用keras lstm写一个带有注意机制的例子,要用keras.Attention
时间: 2023-07-09 07:05:48 浏览: 229
好的,下面是一个使用Keras中的LSTM和注意力机制的示例:
```python
from keras.layers import Input, Dense, LSTM, Bidirectional, Concatenate, dot, Activation
from keras.layers import Dropout, Embedding, Flatten, Layer, Multiply
from keras import Model
from keras import backend as K
class Attention(Layer):
def __init__(self, **kwargs):
super(Attention, self).__init__(**kwargs)
def build(self, input_shape):
self.W = self.add_weight(name="att_weight", shape=(input_shape[-1], 1), initializer="normal")
self.b = self.add_weight(name="att_bias", shape=(input_shape[1], 1), initializer="zeros")
super(Attention, self).build(input_shape)
def call(self, x):
e = K.tanh(K.dot(x, self.W) + self.b)
a = K.softmax(e, axis=1)
output = x * a
return K.sum(output, axis=1)
def compute_output_shape(self, input_shape):
return (input_shape[0], input_shape[-1])
# 定义模型
input_1 = Input(shape=(50,))
embedding_layer = Embedding(input_dim=1000, output_dim=50, input_length=50)(input_1)
lstm_layer = LSTM(64, dropout=0.2, recurrent_dropout=0.2, return_sequences=True)(embedding_layer)
bilstm_layer = Bidirectional(LSTM(32, dropout=0.2, recurrent_dropout=0.2, return_sequences=True))(lstm_layer)
attention_layer = Attention()(bilstm_layer)
dense_layer = Dense(1)(attention_layer)
output = Activation('sigmoid')(dense_layer)
model = Model(inputs=input_1, outputs=output)
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
model.summary()
```
这是一个简单的LSTM模型,其中包含一个双向LSTM层和一个注意力机制层。在这个模型中,我们首先使用Embedding层将输入的整数序列转换为向量序列。然后,我们将这些向量传递到LSTM层中,该层的输出是一个序列。接下来,我们使用双向LSTM层来进一步处理序列,并获得更好的表示。最后,我们使用自定义的Attention层来聚合序列,并生成最终的输出。
阅读全文
相关推荐
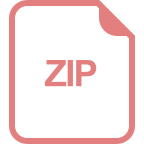
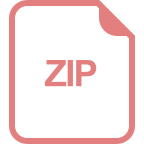

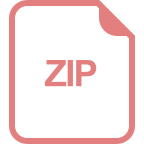













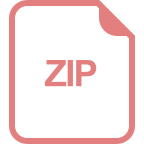