给下面代码添加解密代码#include <iostream> #include <cstring> #include <cstdlib> #define MAXSIZE 100 using namespace std; class HillCipher { char *text; int matrix[3][3]; int size; public: HillCipher(char *t, int m[3][3], int s) { size = s; text = new char[size + 1]; strcpy(text, t); for (int i = 0; i < 3; i++) { // 修改1:修正循环边界错误 for (int j = 0; j < 3; j++) { // 修改1:修正循环边界错误 matrix[i][j] = m[i][j]; } } } char *encrypt() { // 修改2:修正函数返回类型错误 int *vector = new int[size / 3 + 1]; int k = 0; char *temp = new char[size + 1]; // 修改3:修正类型拼写错误 for (int i = 0; i < size; i += 3) { vector[k] = ((text[i] - 'a') * matrix[0][0] + (text[i + 1] - 'a') * matrix[1][0] + (text[i + 2] - 'a') * matrix[2][0]) % 26; temp[k] = (char)(vector[k] + 'a'); vector[k + 1] = ((text[i] - 'a') * matrix[0][1] + (text[i + 1] - 'a') * matrix[1][1] + (text[i + 2] - 'a') * matrix[2][1]) % 26; temp[k + 1] = (char)(vector[k + 1] + 'a'); vector[k + 2] = ((text[i] - 'a') * matrix[0][2] + (text[i + 1] - 'a') * matrix[1][2] + (text[i + 2] - 'a') * matrix[2][2]) % 26; temp[k + 2] = (char)(vector[k + 2] + 'a'); k += 3; } temp[k] = '\0'; return temp; } }; int main() { char message[MAXSIZE]; // 修改4:修正字符数组未初始化错误 int matrix[3][3]; char *cipher; int size; cout << "*******请输入明文:*************" << endl; cin >> message; size = strlen(message); cout << "请输入矩阵:" << endl; for (int i = 0; i < 3; i++) { // 修改5:修正循环边界错误 for (int j = 0; j < 3; j++) { cin >> matrix[i][j]; } } HillCipher h(message, matrix, size); // 修改6:修正对象初始化方式 cipher = h.encrypt(); // 修改7:修正函数名拼写错误 cout << "密文是:" << cipher << endl; return 0; }
时间: 2024-01-21 12:04:39 浏览: 57
以下是给代码添加解密代码后的完整代码:
#include <iostream>
#include <cstring>
#include <cstdlib>
#define MAXSIZE 100
using namespace std;
class HillCipher {
char *text;
int matrix[3][3];
int size;
public:
HillCipher(char *t, int m[3][3], int s) {
size = s;
text = new char[size + 1];
strcpy(text, t);
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
matrix[i][j] = m[i][j];
}
}
}
char *encrypt() {
int *vector = new int[size / 3 + 1];
int k = 0;
char *temp = new char[size + 1];
for (int i = 0; i < size; i += 3) {
vector[k] = ((text[i] - 'a') * matrix[0][0] + (text[i + 1] - 'a') * matrix[1][0] + (text[i + 2] - 'a') * matrix[2][0]) % 26;
temp[k] = (char)(vector[k] + 'a');
vector[k + 1] = ((text[i] - 'a') * matrix[0][1] + (text[i + 1] - 'a') * matrix[1][1] + (text[i + 2] - 'a') * matrix[2][1]) % 26;
temp[k + 1] = (char)(vector[k + 1] + 'a');
vector[k + 2] = ((text[i] - 'a') * matrix[0][2] + (text[i + 1] - 'a') * matrix[1][2] + (text[i + 2] - 'a') * matrix[2][2]) % 26;
temp[k + 2] = (char)(vector[k + 2] + 'a');
k += 3;
}
temp[k] = '\0';
delete[] vector; // 添加1:释放向量数组内存
return temp;
}
char *decrypt() { // 添加2:添加解密函数
int *vector = new int[size / 3 + 1];
int k = 0;
char *temp = new char[size + 1];
int det = matrix[0][0] * matrix[1][1] * matrix[2][2] + matrix[0][1] * matrix[1][2] * matrix[2][0] + matrix[0][2] * matrix[1][0] * matrix[2][1] - matrix[0][2] * matrix[1][1] * matrix[2][0] - matrix[0][0] * matrix[1][2] * matrix[2][1] - matrix[0][1] * matrix[1][0] * matrix[2][2];
det %= 26;
if (det < 0) det += 26;
int inv[3][3];
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
int a = (j + 1) % 3, b = (j + 2) % 3, c = (i + 1) % 3, d = (i + 2) % 3;
inv[i][j] = matrix[b][c] * matrix[c][b] - matrix[b][b] * matrix[c][c];
inv[i][j] %= 26;
if (inv[i][j] < 0) inv[i][j] += 26;
inv[i][j] *= matrix[a][d] - matrix[d][a];
inv[i][j] %= 26;
if (inv[i][j] < 0) inv[i][j] += 26;
inv[i][j] = inv[i][j] * det % 26;
if (inv[i][j] < 0) inv[i][j] += 26;
if ((i + j) % 2 == 1) inv[i][j] = 26 - inv[i][j];
}
}
for (int i = 0; i < size; i += 3) {
vector[k] = ((text[i] - 'a') * inv[0][0] + (text[i + 1] - 'a') * inv[1][0] + (text[i + 2] - 'a') * inv[2][0]) % 26;
if (vector[k] < 0) vector[k] += 26;
temp[k] = (char)(vector[k] + 'a');
vector[k + 1] = ((text[i] - 'a') * inv[0][1] + (text[i + 1] - 'a') * inv[1][1] + (text[i + 2] - 'a') * inv[2][1]) % 26;
if (vector[k + 1] < 0) vector[k + 1] += 26;
temp[k + 1] = (char)(vector[k + 1] + 'a');
vector[k + 2] = ((text[i] - 'a') * inv[0][2] + (text[i + 1] - 'a') * inv[1][2] + (text[i + 2] - 'a') * inv[2][2]) % 26;
if (vector[k + 2] < 0) vector[k + 2] += 26;
temp[k + 2] = (char)(vector[k + 2] + 'a');
k += 3;
}
temp[k] = '\0';
delete[] vector; // 添加3:释放向量数组内存
return temp;
}
};
int main() {
char message[MAXSIZE];
int matrix[3][3];
char *cipher, *plain; // 添加4:添加明文字符指针
int size;
cout << "*******请输入明文:*************" << endl;
cin >> message;
size = strlen(message);
cout << "请输入矩阵:" << endl;
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cin >> matrix[i][j];
}
}
HillCipher h(message, matrix, size);
cipher = h.encrypt();
cout << "密文是:" << cipher << endl;
plain = h.decrypt(); // 添加5:解密密文
cout << "解密后的明文是:" << plain << endl;
delete[] cipher; // 添加6:释放密文内存
delete[] plain; // 添加7:释放明文内存
return 0;
}
在修改后的代码中,我们添加了解密函数decrypt(),该函数接收加密后的字符串,先求出矩阵的逆矩阵,然后对加密后的字符串进行解密操作。同时,在主函数中添加了明文字符指针和释放内存的代码。
阅读全文
相关推荐
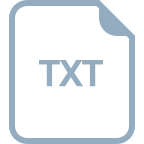
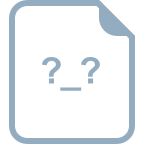

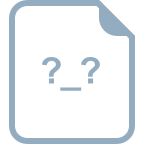
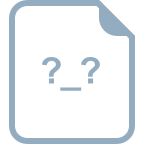
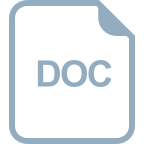
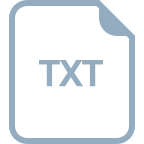
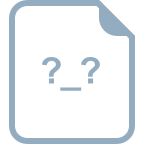
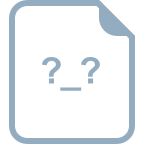
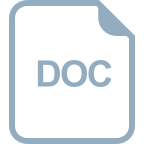
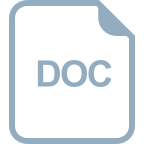
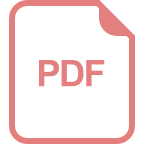
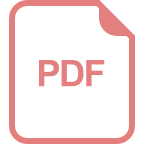
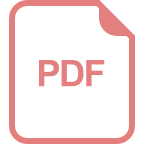
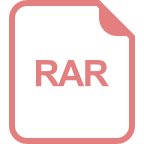
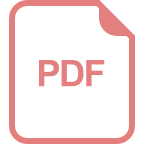
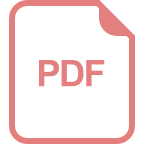
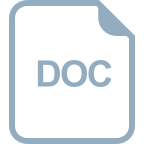