winform中调用摄像头扫描条形码在文本框中,完整代码和组件
时间: 2024-02-22 08:00:09 浏览: 31
以下是一个调用摄像头扫描条形码并在文本框中显示的WinForm应用程序的完整代码和所需组件:
1. 在项目中添加一个PictureBox控件和一个TextBox控件。
2. 在NuGet包管理器中搜索并安装ZXing.Net包。这是一个用于解码条形码的开源库。
3. 将以下代码添加到Form1.cs文件中:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using ZXing;
namespace BarcodeScanner
{
public partial class Form1 : Form
{
private readonly Webcam webcam;
public Form1()
{
InitializeComponent();
// 初始化摄像头
webcam = new Webcam(pictureBox1);
webcam.OnCaptured += Webcam_OnCaptured;
}
private void Form1_Load(object sender, EventArgs e)
{
// 启动摄像头
webcam.Start();
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
// 停用摄像头
webcam.Stop();
}
private void Webcam_OnCaptured(object source, WebcamEventArgs e)
{
// 解码条形码
var barcodeReader = new BarcodeReader();
var result = barcodeReader.Decode((Bitmap)e.Frame.Clone());
if (result != null)
{
// 在文本框中显示条形码内容
textBox1.Invoke(new Action(() => textBox1.Text = result.Text));
}
}
}
}
```
4. 添加一个名为“Webcam”的新类:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
namespace BarcodeScanner
{
public class Webcam
{
private readonly PictureBox pictureBox;
private readonly Timer timer;
public event EventHandler<WebcamEventArgs> OnCaptured;
public Webcam(PictureBox pictureBox)
{
this.pictureBox = pictureBox;
this.timer = new Timer();
this.timer.Tick += Timer_Tick;
this.timer.Interval = 100;
}
public void Start()
{
var devices = new WebCamLib.VideoCaptureDevices();
if (devices.Count == 0)
{
throw new Exception("No webcam found!");
}
var device = devices[0];
device.VideoResolution = device.SupportedVideoResolutions[0];
device.Start();
timer.Start();
}
public void Stop()
{
timer.Stop();
var devices = new WebCamLib.VideoCaptureDevices();
if (devices.Count > 0)
{
devices[0].Stop();
}
}
private void Timer_Tick(object sender, EventArgs e)
{
var devices = new WebCamLib.VideoCaptureDevices();
if (devices.Count > 0)
{
var device = devices[0];
var frame = device.GetCurrentFrame();
pictureBox.Image = frame;
OnCaptured?.Invoke(this, new WebcamEventArgs(frame));
}
}
}
public class WebcamEventArgs : EventArgs
{
public Bitmap Frame { get; }
public WebcamEventArgs(Bitmap frame)
{
Frame = frame;
}
}
}
```
5. 运行应用程序,将摄像头对准条形码,扫描后条形码内容将显示在文本框中。
阅读全文
相关推荐
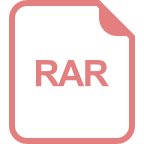





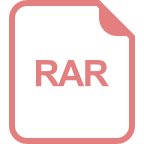
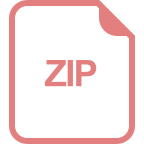
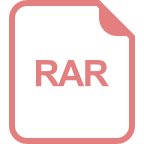
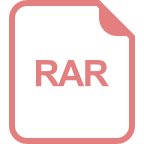
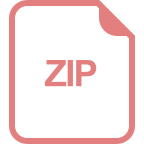
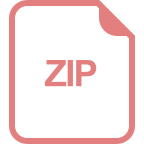
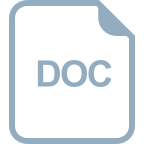


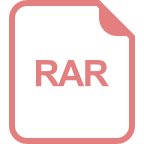