基于stm32单片机关于LCD1602实时显示DS1820温度传感器的相关程序
时间: 2023-09-03 20:05:54 浏览: 55
以下是基于STM32单片机的LCD1602实时显示DS1820温度传感器的程序:
```c
#include "stm32f10x.h"
#include "delay.h"
#include "lcd1602.h"
#include "ds18b20.h"
int main(void)
{
float temp;
char str[16];
// 初始化延时函数
Delay_Init();
// 初始化LCD1602
LCD1602_Init();
// 初始化DS18B20温度传感器
DS18B20_Init();
while(1)
{
// 获取DS18B20温度传感器的温度值
temp = DS18B20_GetTemp();
// 将温度值转换成字符串
sprintf(str, "Temp: %.1f C", temp);
// 在LCD1602上显示温度值
LCD1602_DisplayStr(0, 0, str);
// 延时1秒
Delay_ms(1000);
}
}
```
其中需要使用到延时函数、LCD1602驱动以及DS18B20温度传感器驱动。请确保这些驱动已经正确地引入到程序中。
相关问题
基于stm32单片机和1602液晶显示的DS1820的程序编写的
以下是基于STM32单片机和1602液晶显示的DS1820程序编写的基本步骤:
1. 确定STM32单片机的型号和引脚布局,以及DS1820温度传感器的引脚布局。
2. 配置STM32单片机的GPIO口,将DS1820温度传感器的引脚与对应的GPIO口相连。
3. 初始化DS1820温度传感器,发送初始化序列,等待DS1820的响应信号。
4. 发送读取温度命令,等待DS1820的响应信号。
5. 从DS1820读取温度数据,并进行温度转换和校准。
6. 将温度数据通过USART串口输出到PC端,或者通过LCD1602显示模块显示在1602液晶屏幕上。
下面是一个基于STM32F103C8T6单片机和DS1820温度传感器的程序示例:
```c
#include "stm32f10x.h"
#include "delay.h"
#define DS1820_GPIO_PORT GPIOB
#define DS1820_GPIO_PIN GPIO_Pin_12
void DS1820_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = DS1820_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DS1820_GPIO_PORT, &GPIO_InitStructure);
GPIO_SetBits(DS1820_GPIO_PORT, DS1820_GPIO_PIN);
delay_us(10);
GPIO_ResetBits(DS1820_GPIO_PORT, DS1820_GPIO_PIN);
delay_us(500);
GPIO_SetBits(DS1820_GPIO_PORT, DS1820_GPIO_PIN);
delay_us(100);
}
void DS1820_WriteBit(uint8_t bit)
{
GPIO_ResetBits(DS1820_GPIO_PORT, DS1820_GPIO_PIN);
delay_us(10);
if (bit) GPIO_SetBits(DS1820_GPIO_PORT, DS1820_GPIO_PIN);
delay_us(90);
GPIO_SetBits(DS1820_GPIO_PORT, DS1820_GPIO_PIN);
delay_us(10);
}
uint8_t DS1820_ReadBit(void)
{
uint8_t bit = 0;
GPIO_ResetBits(DS1820_GPIO_PORT, DS1820_GPIO_PIN);
delay_us(2);
GPIO_SetBits(DS1820_GPIO_PORT, DS1820_GPIO_PIN);
delay_us(8);
if (GPIO_ReadInputDataBit(DS1820_GPIO_PORT, DS1820_GPIO_PIN)) bit = 1;
delay_us(80);
return bit;
}
void DS1820_WriteByte(uint8_t byte)
{
uint8_t i;
for (i = 0; i < 8; i++) {
DS1820_WriteBit(byte & 0x01);
byte >>= 1;
}
}
uint8_t DS1820_ReadByte(void)
{
uint8_t i, byte = 0;
for (i = 0; i < 8; i++) {
byte >>= 1;
if (DS1820_ReadBit()) byte |= 0x80;
}
return byte;
}
void DS1820_Start(void)
{
DS1820_Init();
DS1820_WriteByte(0xCC); // Skip ROM command
DS1820_WriteByte(0x44); // Start conversion command
}
float DS1820_ReadTemperature(void)
{
uint8_t temperature[2];
float temp;
DS1820_Init();
DS1820_WriteByte(0xCC); // Skip ROM command
DS1820_WriteByte(0xBE); // Read scratchpad command
temperature[0] = DS1820_ReadByte();
temperature[1] = DS1820_ReadByte();
temp = temperature[0] | (temperature[1] << 8);
temp *= 0.0625;
return temp;
}
int main(void)
{
float temp;
char str[16];
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO | RCC_APB2Periph_USART1, ENABLE);
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_Cmd(USART1, ENABLE);
while (1) {
DS1820_Start();
temp = DS1820_ReadTemperature();
sprintf(str, "Temperature: %.2fC\r\n", temp);
USART_SendString(USART1, str);
delay_ms(1000);
}
}
```
在上述程序中,我们通过USART串口将温度数据输出到PC端,也可以通过LCD1602显示模块将温度数据显示在液晶屏幕上。需要注意的是,不同型号的STM32单片机引脚布局可能会有所不同,需要根据具体型号进行调整。
在stm32单片机用LCD1602和DS18B20显示温度的代码
以下是在STM32单片机使用LCD1602和DS18B20显示温度的代码:
```
#include "stm32f10x.h"
#include "delay.h"
#include "lcd1602.h"
#include "ds18b20.h"
int main(void)
{
uint8_t temperature_string[7] = {0}; // 存储温度值转换为字符串后的结果
// 初始化LCD1602显示屏
lcd1602_init();
// 初始化DS18B20温度传感器
ds18b20_init(GPIOB, GPIO_Pin_8);
// 无限循环
while(1)
{
float temperature = ds18b20_read_temp(GPIOB, GPIO_Pin_8); // 读取温度
// 将温度转换为带一位小数的字符串形式
int integer_part = (int)temperature;
int decimal_part = (int)(temperature * 10) % 10;
sprintf((char*)temperature_string, "%d.%dC", integer_part, decimal_part);
// 在LCD1602显示屏上显示温度
lcd1602_write_string(0, 0, "Temperature:");
lcd1602_write_string(0, 1, (char*)temperature_string);
// 延迟一段时间再进行下一次循环,避免过于频繁的读取温度传感器
delay_ms(1000);
}
}
```
其中,`delay.h`和`delay.c`文件可以用外部提供的延时库,也可以自行编写。`lcd1602.h`和`lcd1602.c`文件是LCD1602显示屏的驱动代码,提供了一些常用的函数,如`lcd1602_init()`用于初始化LCD1602显示屏,`lcd1602_write_string()`用于在指定位置上显示字符串。`ds18b20.h`和`ds18b20.c`文件是DS18B20温度传感器的驱动代码,提供了一些常用的函数,如`ds18b20_init()`用于初始化DS18B20温度传感器,`ds18b20_read_temp()`用于读取温度。在以上代码中,GPIOB的第8个引脚分别连接到DS18B20温度传感器的DATA引脚,用于获取温度值并显示在LCD1602显示屏上。
相关推荐
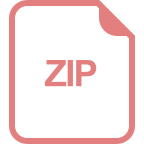
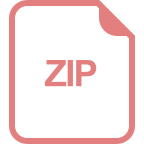
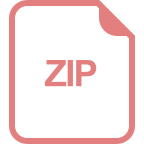
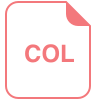
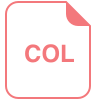
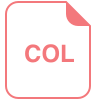
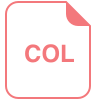
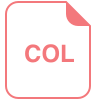







