python单例模式对象
时间: 2023-11-22 08:50:55 浏览: 102
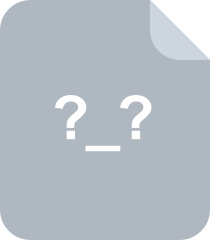
python 设计模式之单例模式
Python中实现单例模式有多种方法,以下是两种常见的实现方式:
1.使用元类实现单例模式:
在元类中创建 __call__ 方法,该方法会在 class A 和 B 创建对象时调用,在该方法中会调用 __new__ 和 __init__ 方法,创建完对象后,再将该对象放在类变量 _instance 中,和在__new__中实现单例的方法一样。
```python
from typing import Any
class Singleton(type):
def __call__(self, *args: Any, **kwds: Any) -> Any:
if not hasattr(self, "_instance"):
self._instance = super(Singleton, self).__call__(*args, **kwds)
return self._instance
class A(metaclass=Singleton):
pass
class B(metaclass=Singleton):
pass
```
2.使用装饰器实现单例模式:
通过将所有的单例对象保存在装饰器的 _instance 字典中,以类为 key,对象为 value 进行存储。
```python
def Singleton(cls):
_instance = {}
def _singleton(*args, **kargs):
if cls not in _instance:
_instance[cls] = cls(*args, **kargs)
return _instance[cls]
return _singleton
@Singleton
class A:
pass
@Singleton
class B:
pass
```
阅读全文
相关推荐






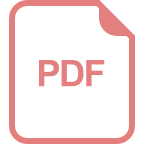
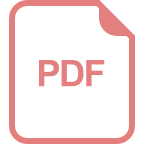
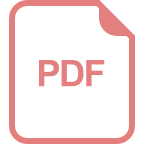
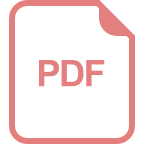
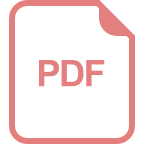
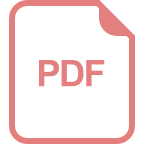
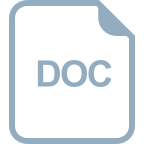
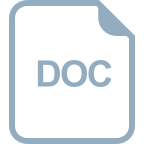
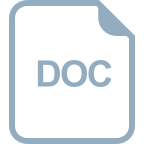

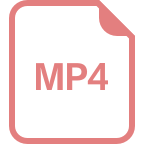