vue3 three.js 卫星地图
时间: 2023-11-12 18:08:11 浏览: 89
可以使用 Three.js 来创建卫星地图,具体步骤如下:
1. 获取卫星地图的纹理贴图,可以从 NASA 的网站上下载。
2. 使用 Three.js 中的 TextureLoader 加载纹理贴图。
3. 创建一个球体作为地球,并将纹理贴图应用到球体上。
4. 创建一个相机和一个场景,并将球体添加到场景中。
5. 使用 OrbitControls 控制相机的位置和旋转,使其能够围绕球体旋转。
6. 使用渲染器将场景渲染到页面上。
相关问题
vue+three.js3d地图
Vue和Three.js是两个不同的技术,Vue是一个前端框架,而Three.js是一个基于WebGL的JavaScript库,用于创建3D图形。使用Vue和Three.js可以很方便地实现3D地图场景的展示。
在使用Vue和Three.js创建3D地图时,首先需要设置场景(Scene)、相机(Camera)、光源(Lighting)、对象(Object)等元素,并将它们组合在一起。可以使用Vue的组件化方式来构建这些元素,然后将它们添加到场景中。在设置相机时,需要考虑到相机的位置、方向、FoV等参数,以便实现视角的变换和缩放等操作。
当创建好场景、相机、光源和对象后,可以使用Three.js提供的材质(Material)、纹理(Texture)、几何体(Geometry)等API,来实现对象的样式和效果。例如,可以使用纹理图片来为建筑物、地面等对象添加贴图效果,也可以使用材质来控制对象的表面和反射等特性。
除了基础的场景元素和对象设置外,还可以使用Three.js提供的控制器(Controller)来实现用户交互。例如,可以使用鼠标控制器(OrbitControls)来实现用户通过鼠标操作旋转和缩放场景,或使用触摸控制器(TouchControls)来实现在移动设备上的交互。
最后需要注意的是,3D地图的数据源通常比较大,需要进行优化和压缩。可以使用Vue和Three.js提供的工具和插件,来实现数据的处理和性能的优化。例如,可以使用Vue的懒加载和异步组件,来减少初始化时间和提高页面加载速度;也可以使用Three.js的GeometryUtils和TextureLoader等API,来优化数据加载和渲染性能。
总之,使用Vue和Three.js可以很方便地实现3D地图场景的展示,并且可以通过组件化与API的方式实现更加灵活和丰富的效果。
vue3 three.js
Vue3是一个流行的JavaScript框架,而Three.js是一个用于创建和展示3D模型和动画的轻量级跨平台JavaScript库。在Vue3项目中使用Three.js可以通过以下步骤进行:
1. 首先,下载和安装Three.js库。你可以在Vue3项目中使用npm或者yarn来安装Three.js库。
2. 创建一个Vue组件,比如myThree.vue,用于展示Three.js场景和模型。在这个组件中,你可以引入Three.js库并创建一个场景、物体、光源和相机。
3. 在Vue组件中使用Three.js的类来创建和操作Three.js对象。你可以使用reactive函数来创建一个响应式对象,比如`const three = reactive({ base3d: new Base3D("#my_parking", 45, 0.1, 500) })`。这个对象可以用来管理Three.js场景和模型的状态。
4. 在Vue组件的模板中,使用canvas或者其他HTML元素来展示Three.js场景和模型。你可以使用v-for指令来渲染多个模型。
总结起来,使用Vue3和Three.js可以在Vue项目中创建和展示3D模型和动画。你可以通过下载和安装Three.js库,创建Vue组件并使用Three.js的类来操作Three.js对象,最后在模板中展示Three.js场景和模型。
相关推荐
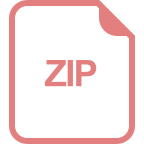
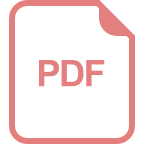












