使用svm训练KDEF
时间: 2024-10-08 11:16:02 浏览: 22
SVM(支持向量机)是一种监督学习算法,在情感分析、文本分类等任务中有广泛应用。当你想要利用KDEF(Karolinska Directed Emotional Faces)数据集训练SVM模型时,首先要了解以下几个步骤:
1. **数据准备**:KDEF是一个包含不同表情的人脸数据库,用于研究面部表情与情绪之间的关系。你需要将人脸图像与其对应的标签(如快乐、悲伤、愤怒等基本情绪类别)对应起来。
2. **特征提取**:从人脸图像中提取有用的特征,这可以是基于视觉的特征(例如Haar特征、HOG、LBP等),也可以是深度学习特征(如卷积神经网络的中间层输出)。
3. **预处理**:对提取的特征进行归一化、降维或标准化,以便于SVM的处理。
4. **划分数据集**:通常会将数据集分为训练集、验证集和测试集,以便评估模型性能。
5. **训练模型**:使用Scikit-learn或其他机器学习库提供的SVM类(如`sklearn.svm.SVC`或`sklearn.linear_model.SGDClassifier`),通过训练集来构建模型,调整参数如C值(惩罚项)、核函数等。
6. **交叉验证**:为了防止过拟合并优化参数,可能需要进行交叉验证,如k折交叉验证。
7. **评估与优化**:使用验证集评估模型的表现,如准确率、精确率、召回率等指标。如果结果不佳,可以尝试调整模型参数或改变特征选择。
8. **测试阶段**:最后,使用测试集验证模型在未见过的数据上的泛化能力。
相关问题
用Python实现1.熟悉支持向量机SVM (Support Vector Machine)模型分类算法的使用。 2.利用高斯核作为核函数训练模型。 3.保存并调用训练模型并预测自己给出样例的类别。 4.实现SVM分类的可视化。
好的,您的问题是如何用Python实现支持向量机SVM模型分类算法的使用,利用高斯核作为核函数训练模型,并保存并调用训练模型并预测自己给出样例的类别,最后实现SVM分类的可视化。
以下是一些基本的代码实现步骤:
1.导入必要的库
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn import svm, datasets
```
2.导入数据
本例中,我们将使用鸢尾花数据集(iris dataset)。
```python
iris = datasets.load_iris()
X = iris.data[:, :2]
y = iris.target
```
3.将数据集分成训练集和测试集
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=0)
```
4.训练SVM模型
```python
C = 1.0 # SVM regularization parameter
svc = svm.SVC(kernel='rbf', gamma=0.7, C=C).fit(X_train, y_train)
```
在这里,我们使用高斯核函数(rbf)作为核函数。参数gamma控制高斯核的宽度。
5.预测测试集的类别
```python
y_pred = svc.predict(X_test)
```
6.评估准确性
```python
from sklearn.metrics import accuracy_score
accuracy_score(y_test, y_pred)
```
7.保存模型
```python
import joblib
joblib.dump(svc, 'svm_model.pkl')
```
8.加载模型并进行预测
```python
clf = joblib.load('svm_model.pkl')
clf.predict([[5.1, 3.5]])
```
9.可视化SVM分类结果
```python
def plot_svc_decision_function(model, ax=None, plot_support=True):
if ax is None:
ax = plt.gca()
xlim = ax.get_xlim()
ylim = ax.get_ylim()
# create grid to evaluate model
x = np.linspace(xlim[0], xlim[1], 30)
y = np.linspace(ylim[0], ylim[1], 30)
Y, X = np.meshgrid(y, x)
xy = np.vstack([X.ravel(), Y.ravel()]).T
P = model.decision_function(xy).reshape(X.shape)
# plot decision boundary and margins
ax.contour(X, Y, P, colors='k', levels=[-1, 0, 1], alpha=0.5,
linestyles=['--', '-', '--'])
# plot support vectors
if plot_support:
ax.scatter(model.support_vectors_[:, 0],
model.support_vectors_[:, 1],
s=300, linewidth=1, facecolors='none')
ax.set_xlim(xlim)
ax.set_ylim(ylim)
plt.scatter(X[:, 0], X[:, 1], c=y, cmap=plt.cm.Paired)
plot_svc_decision_function(svc)
plt.show()
```
这里我们定义了一个绘制SVM分类结果的函数plot_svc_decision_function。它可以绘制决策边界和支持向量。
希望这可以帮助您实现您的目标。
使用python实现非线性SVM算法
非线性SVM算法可以使用核函数来解决非线性分类问题。下面是使用Python实现非线性SVM算法的步骤:
1. 导入需要的库和数据集
```python
from sklearn.datasets import make_circles
import matplotlib.pyplot as plt
import numpy as np
X, y = make_circles(n_samples=100, noise=0.1, factor=0.1, random_state=42)
```
2. 可视化数据集
```python
plt.scatter(X[:, 0], X[:, 1], c=y)
plt.show()
```
3. 定义核函数
这里我们使用径向基函数(RBF)作为核函数。
```python
def rbf_kernel(x1, x2, gamma):
return np.exp(-gamma * np.linalg.norm(x1 - x2) ** 2)
```
4. 定义非线性SVM分类器
```python
class NonlinearSVM:
def __init__(self, gamma=1.0):
self.gamma = gamma
def fit(self, X, y):
n_samples, n_features = X.shape
# 计算Gram矩阵
K = np.zeros((n_samples, n_samples))
for i in range(n_samples):
for j in range(n_samples):
K[i, j] = rbf_kernel(X[i], X[j], self.gamma)
# 定义二次规划问题
from cvxopt import matrix, solvers
P = matrix(np.outer(y, y) * K)
q = matrix(np.ones(n_samples) * -1)
A = matrix(y, (1, n_samples))
b = matrix(0.0)
G = matrix(np.diag(np.ones(n_samples) * -1))
h = matrix(np.zeros(n_samples))
# 求解二次规划问题
solvers.options['show_progress'] = False
sol = solvers.qp(P, q, G, h, A, b)
alphas = np.array(sol['x']).reshape(n_samples)
# 提取支持向量
threshold = 1e-5
sv_indices = alphas > threshold
self.support_vectors = X[sv_indices]
self.support_vector_labels = y[sv_indices]
self.support_vector_alphas = alphas[sv_indices]
# 计算偏置项
self.b = np.mean(
[y_k - self.predict(x_k, self.support_vectors, self.support_vector_labels, self.support_vector_alphas)
for (y_k, x_k) in zip(self.support_vector_labels, self.support_vectors)])
def predict(self, x, support_vectors, support_vector_labels, support_vector_alphas):
y_predict = 0
for i in range(len(support_vector_labels)):
y_predict += support_vector_alphas[i] * support_vector_labels[i] * rbf_kernel(x, support_vectors[i], self.gamma)
y_predict += self.b
return np.sign(y_predict)
```
5. 训练模型并可视化决策边界
```python
clf = NonlinearSVM(gamma=1.0)
clf.fit(X, y)
# 可视化决策边界
def plot_contours(ax, clf, xx, yy, **params):
Z = clf.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
out = ax.contourf(xx, yy, Z, **params)
return out
xx, yy = np.meshgrid(np.linspace(-1.5, 1.5, 100), np.linspace(-1.5, 1.5, 100))
fig, ax = plt.subplots()
plot_contours(ax, clf, xx, yy, cmap=plt.cm.coolwarm, alpha=0.8)
plt.scatter(X[:, 0], X[:, 1], c=y)
plt.show()
```
完整代码如下:
```python
from sklearn.datasets import make_circles
import matplotlib.pyplot as plt
import numpy as np
X, y = make_circles(n_samples=100, noise=0.1, factor=0.1, random_state=42)
plt.scatter(X[:, 0], X[:, 1], c=y)
plt.show()
def rbf_kernel(x1, x2, gamma):
return np.exp(-gamma * np.linalg.norm(x1 - x2) ** 2)
class NonlinearSVM:
def __init__(self, gamma=1.0):
self.gamma = gamma
def fit(self, X, y):
n_samples, n_features = X.shape
# 计算Gram矩阵
K = np.zeros((n_samples, n_samples))
for i in range(n_samples):
for j in range(n_samples):
K[i, j] = rbf_kernel(X[i], X[j], self.gamma)
# 定义二次规划问题
from cvxopt import matrix, solvers
P = matrix(np.outer(y, y) * K)
q = matrix(np.ones(n_samples) * -1)
A = matrix(y, (1, n_samples))
b = matrix(0.0)
G = matrix(np.diag(np.ones(n_samples) * -1))
h = matrix(np.zeros(n_samples))
# 求解二次规划问题
solvers.options['show_progress'] = False
sol = solvers.qp(P, q, G, h, A, b)
alphas = np.array(sol['x']).reshape(n_samples)
# 提取支持向量
threshold = 1e-5
sv_indices = alphas > threshold
self.support_vectors = X[sv_indices]
self.support_vector_labels = y[sv_indices]
self.support_vector_alphas = alphas[sv_indices]
# 计算偏置项
self.b = np.mean(
[y_k - self.predict(x_k, self.support_vectors, self.support_vector_labels, self.support_vector_alphas)
for (y_k, x_k) in zip(self.support_vector_labels, self.support_vectors)])
def predict(self, x, support_vectors, support_vector_labels, support_vector_alphas):
y_predict = 0
for i in range(len(support_vector_labels)):
y_predict += support_vector_alphas[i] * support_vector_labels[i] * rbf_kernel(x, support_vectors[i], self.gamma)
y_predict += self.b
return np.sign(y_predict)
clf = NonlinearSVM(gamma=1.0)
clf.fit(X, y)
# 可视化决策边界
def plot_contours(ax, clf, xx, yy, **params):
Z = clf.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
out = ax.contourf(xx, yy, Z, **params)
return out
xx, yy = np.meshgrid(np.linspace(-1.5, 1.5, 100), np.linspace(-1.5, 1.5, 100))
fig, ax = plt.subplots()
plot_contours(ax, clf, xx, yy, cmap=plt.cm.coolwarm, alpha=0.8)
plt.scatter(X[:, 0], X[:, 1], c=y)
plt.show()
```
阅读全文
相关推荐
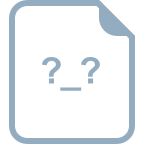
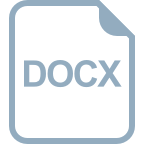
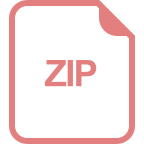
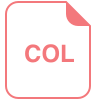













