unityc#完整脚本中如何让sphere引用绑定在cube的脚本
时间: 2024-09-24 16:26:37 浏览: 33
在Unity C#中,如果你想让Sphere引用并执行 Cube 上绑定的脚本中的某些功能,可以使用继承或委托的方式来实现。假设Cube上的脚本名为`CubeScript`,并且它有一个公开的方法`ChangeSphereColorToYellow()`,你可以这样做:
首先,为Sphere创建一个新脚本`SphereScriptReference`,让它继承自`CubeScript`或者简单地添加对`CubeScript`方法的调用:
```csharp
using UnityEngine;
public class SphereScriptReference : CubeScript
{
public override void CustomFunctionality() // 如果立方体脚本中有CustomFunctionality方法
{
base.CustomFunctionality(); // 先调用父类方法
// 调用立方体脚本改变颜色的方法
ChangeSphereColorToYellow();
}
}
```
然后,将此`SphereScriptReference`脚本附加到Sphere上。这样,每当`CubeScript`的相应方法被调用时,`SphereScriptReference`也会同步执行。
另一种方法是使用委托(Delegates):
```csharp
using UnityEngine;
public class CubeScript
{
public delegate void ChangeColorAction();
public event ChangeColorAction ChangeColorEvent;
public void ChangeSphereColorToYellow()
{
ChangeColorEvent?.Invoke();
}
}
public class SphereScriptReference : MonoBehaviour
{
private CubeScript cubeScript;
void Start()
{
cubeScript = GetComponent<CubeScript>();
if (cubeScript != null)
{
cubeScript.ChangeColorEvent += ChangeColorHandler;
}
}
void OnDestroy()
{
cubeScript?.ChangeColorEvent -= ChangeColorHandler;
}
private void ChangeColorHandler()
{
GetComponent<Renderer>().color = Color.yellow;
}
}
```
在这个例子中,`SphereScriptReference`订阅了`CubeScript`的`ChangeColorEvent`,并在接到通知时改变Sphere的颜色。
阅读全文
相关推荐
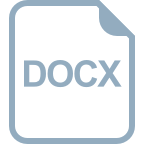
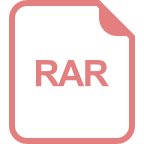
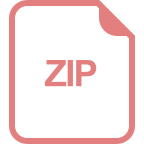

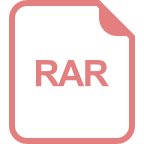
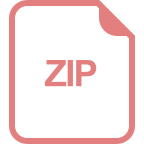
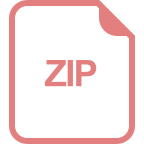
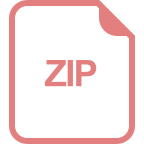
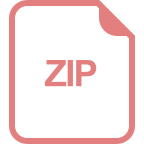
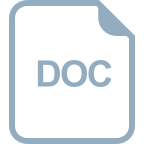







