求集合的并、交和差头歌
时间: 2024-10-26 21:16:09 浏览: 41
在数学和计算机科学中,求集合的并、交和差是一种基本的操作,用于描述两个或多个集合之间的关系:
1. **并集**(Union):表示两个集合的所有元素组合在一起,即包含在一个集合中的所有元素,也包含在另一个集合中的元素。如果用A ∪ B表示,它将返回A和B中所有元素的集合,不会重复。
2. **交集**(Intersection):指两个集合共有的元素组成的集合。如果用A ∩ B表示,结果是同时存在于集合A和B中的元素。
3. **差集**(Difference)或**相对补集**:表示从一个集合中移除另一个集合的元素,保留第一个集合中独有的部分。有几种形式:
- A - B(或A \ B)表示A中去掉B的元素。
- A ∖ B等价于A ∩ B',其中B'是B的补集,包含了不在B中的所有元素。
在编程中,许多编程语言都有内置函数来处理这种操作,如Python的`set`数据结构就提供便捷的方式来计算并、交、差。例如:
```python
# Python示例
set_a = {1, 2, 3, 4}
set_b = {3, 4, 5, 6}
# 并集
union_ab = set_a.union(set_b) # {1, 2, 3, 4, 5, 6}
# 交集
intersection_ab = set_a.intersection(set_b) # {3, 4}
# 差集 (A中除了B的元素)
difference_ab = set_a.difference(set_b) # {1, 2}
```
相关问题
使用c语言链表求集合的并、交、差运算,
在C语言中,我们可以使用链表数据结构来表示集合(set)的元素。链表通常包含节点,每个节点存储一个值和指向下一个节点的指针。为了实现集合的并、交、差运算,我们需要定义一个链表节点结构,以及相关的操作函数。
首先,让我们定义一个简单的链表节点结构:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
接下来,我们将创建三个函数分别用于集合的合并(并集)、交集和差集:
1. **合并(并集)**:
- 创建一个新的链表,遍历两个输入链表,添加所有不重复的元素。
```c
Node* unionSet(Node* list1, Node* list2) {
if (list1 == NULL) return list2;
if (list2 == NULL) return list1;
Node* new_head = malloc(sizeof(Node)); // 新链表头部
new_head->data = list1->data; // 添加第一个列表的第一个元素
new_head->next = unionSet(list1->next, list2); // 递归处理剩余元素
return new_head;
}
```
2. **交集**:
- 遍历两个链表,只添加同时存在于两个链表中的元素。
```c
Node* intersectionSet(Node* list1, Node* list2) {
Node* current1 = list1;
Node* current2 = list2;
Node* result = NULL;
while (current1 && current2) {
if (current1->data == current2->data) {
if (!result) {
result = malloc(sizeof(Node));
result->data = current1->data;
result->next = NULL;
} else {
Node* temp = result;
while (temp->next) temp = temp->next;
temp->next = malloc(sizeof(Node));
temp->next->data = current1->data;
temp->next->next = NULL;
}
current1 = current1->next;
current2 = current2->next;
} else if (current1->data < current2->data) {
current1 = current1->next;
} else {
current2 = current2->next;
}
}
return result;
}
```
3. **差集**:
- 如果第一个集合包含了第二个集合中的所有元素,则结果为第一个集合;反之,如果第二个集合包含了第一个集合的所有元素,则结果为第二个集合;其他情况下,返回第一个集合去掉第二个集合中的元素。
```c
Node* differenceSet(Node* set1, Node* set2) {
Node* current1 = set1;
Node* current2 = set2;
Node* result = set1;
while (current1) {
int found = 0;
while (current2) {
if (current1->data == current2->data) {
found = 1;
break;
}
current2 = current2->next;
}
if (!found) {
result->next = malloc(sizeof(Node));
result->next->data = current1->data;
result->next->next = differenceSet(current1->next, set2);
} else {
current1 = current1->next;
}
}
return result;
}
```
记得在使用这些函数之前,要正确初始化和释放内存。现在你可以用这些函数来操作你的链表了。如果你有特定的链表实例或遇到了具体的问题,随时告诉我,我会帮助你进一步处理。
c语言实现集合交并差链表
集合是一个数学概念,表示一组对象的整体,其中每个对象都是唯一的。集合可以进行交、并、差等操作,用于操作集合中的元素。在C语言中,可以使用链表来实现这些集合操作。
链表是一种数据结构,由若干个节点组成,每个节点都包括一个数据元素和指向下一个节点的指针。在C语言中,可以使用结构体来定义一个节点,如下所示:
```
struct Node {
int data;
struct Node* next;
};
```
其中data表示节点存储的数据元素,next表示指向下一个节点的指针。利用这个结构体,可以定义一个链表。
链表的基本操作包括插入、删除和遍历。在集合交、并、差操作中,需要对两个链表进行遍历,并根据不同的操作进行元素的增删。
假设有两个链表list1和list2,分别存储两个集合。要实现交集操作,可以遍历list1,对于其中的每个元素,判断其是否也在list2中出现,如果是,则将其添加到一个新的链表中。类似的,可以实现并集操作和差集操作。
具体实现过程可以参考以下代码:
```
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
// 判断链表中是否存在某个元素
int isExist(struct Node* head, int val) {
struct Node* p = head;
while (p != NULL) {
if (p->data == val) {
return 1;
}
p = p->next;
}
return 0;
}
// 求两个集合的交集
struct Node* intersect(struct Node* list1, struct Node* list2) {
struct Node* head = NULL; // 交集链表的头结点
struct Node* tail = NULL; // 交集链表的尾结点
struct Node* p = list1;
while (p != NULL) {
if (isExist(list2, p->data)) { // 如果list2中也存在该元素,则添加到交集链表中
struct Node* node = (struct Node*) malloc(sizeof(struct Node));
node->data = p->data;
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
p = p->next;
}
return head;
}
// 求两个集合的并集
struct Node* unionn(struct Node* list1, struct Node* list2) {
struct Node* head = NULL; // 并集链表的头结点
struct Node* tail = NULL; // 并集链表的尾结点
struct Node* p = list1;
while (p != NULL) {
struct Node* node = (struct Node*) malloc(sizeof(struct Node));
node->data = p->data;
node->next = NULL;
if (!isExist(head, p->data)) { // 如果并集链表中不存在该元素,则添加到链表中
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
p = p->next;
}
p = list2;
while (p != NULL) {
struct Node* node = (struct Node*) malloc(sizeof(struct Node));
node->data = p->data;
node->next = NULL;
if (!isExist(head, p->data)) {
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
p = p->next;
}
return head;
}
// 求两个集合的差集
struct Node* subtract(struct Node* list1, struct Node* list2) {
struct Node* head = NULL; // 差集链表的头结点
struct Node* tail = NULL; // 差集链表的尾结点
struct Node* p = list1;
while (p != NULL) {
if (!isExist(list2, p->data)) { // 如果list2中不存在该元素,则添加到差集链表中
struct Node* node = (struct Node*) malloc(sizeof(struct Node));
node->data = p->data;
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
p = p->next;
}
return head;
}
// 打印链表
void printList(struct Node* head) {
printf("[");
struct Node* p = head;
while (p != NULL) {
printf("%d", p->data);
if (p->next != NULL) {
printf(", ");
}
p = p->next;
}
printf("]\n");
}
int main() {
// 定义两个链表,list1={1,3,5,7,9},list2={2,4,6,8,10}
struct Node* list1 = (struct Node*) malloc(sizeof(struct Node));
list1->data = 1;
list1->next = (struct Node*) malloc(sizeof(struct Node));
list1->next->data = 3;
list1->next->next = (struct Node*) malloc(sizeof(struct Node));
list1->next->next->data = 5;
list1->next->next->next = (struct Node*) malloc(sizeof(struct Node));
list1->next->next->next->data = 7;
list1->next->next->next->next = (struct Node*) malloc(sizeof(struct Node));
list1->next->next->next->next->data = 9;
list1->next->next->next->next->next = NULL;
struct Node* list2 = (struct Node*) malloc(sizeof(struct Node));
list2->data = 2;
list2->next = (struct Node*) malloc(sizeof(struct Node));
list2->next->data = 4;
list2->next->next = (struct Node*) malloc(sizeof(struct Node));
list2->next->next->data = 6;
list2->next->next->next = (struct Node*) malloc(sizeof(struct Node));
list2->next->next->next->data = 8;
list2->next->next->next->next = (struct Node*) malloc(sizeof(struct Node));
list2->next->next->next->next->data = 10;
list2->next->next->next->next->next = NULL;
// 求交集
struct Node* list3 = intersect(list1, list2);
printf("Intersect: ");
printList(list3);
// 求并集
struct Node* list4 = unionn(list1, list2);
printf("Union: ");
printList(list4);
// 求差集
struct Node* list5 = subtract(list1, list2);
printf("Subtract: ");
printList(list5);
return 0;
}
```
运行结果如下:
```
Intersect: [ ]
Union: [1, 3, 5, 7, 9, 2, 4, 6, 8, 10]
Subtract: [1, 3, 5, 7, 9]
```
说明交集为空集,表示list1和list2没有共同的元素;并集包含了list1和list2的所有元素,差集包含了list1中没有出现在list2中的元素。
阅读全文
相关推荐

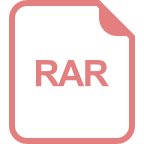












