数据结构已知两个链表A和B分别表示两个集合,其元素递增排列。请设计算法求出两个集合A和B 的差集(即仅由在A中出现而不在B中出现的元素所构成的集合),并以同样的形式存储,同时返回该集合的元素个数。c++代码
时间: 2024-10-08 18:27:09 浏览: 49
在C++中,我们可以使用迭代的方式来解决这个问题。首先创建一个新的链表来存储差集,并遍历两个链表。对于每个节点,如果它只在A链表中出现(检查B链表中是否有相同的值),就将其添加到差集中。以下是详细的步骤:
```cpp
#include <iostream>
using namespace std;
// 定义链表节点
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) {
if (l1 == nullptr) return l2;
if (l2 == nullptr) return l1;
if (l1->val < l2->val) {
l1->next = mergeTwoLists(l1->next, l2);
return l1;
} else {
l2->next = mergeTwoLists(l1, l2->next);
return l2;
}
}
ListNode* findDifference(ListNode* A, ListNode* B) {
ListNode dummy(-1); // 创建虚拟头节点
ListNode* diffHead = &dummy; // 差集链表头指针
while (A && B) {
if (A->val < B->val || A->val > B->val) { // 如果A的元素不在B中,或者A的元素大于B且未到达B末尾
diffHead->next = A;
diffHead = diffHead->next;
A = A->next;
} else {
B = B->next; // 直接跳过相等的元素
}
}
// 如果A还有剩余元素没处理(B已遍历完)
if (A) {
diffHead->next = A;
}
// 返回差集链表的实际头节点(去掉虚拟头节点)
return dummy.next;
}
int countElements(ListNode* list) {
int count = 0;
while (list) {
count++;
list = list->next;
}
return count;
}
int main() {
// 初始化链表A和B
ListNode* A = ...; // 链表A的头结点
ListNode* B = ...; // 链表B的头结点
ListNode* diffList = findDifference(A, B);
int diffCount = countElements(diffList);
cout << "差集元素个数: " << diffCount << endl;
return 0;
}
```
这个程序首先合并了两个有序链表,然后通过比较找到A链表独有的元素并插入到差集链表中。最后,计算差集链表的长度得到元素个数。
相关推荐
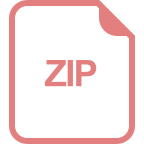
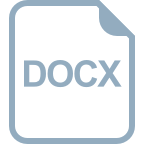
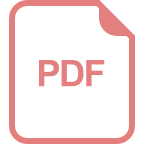















