应用密码学 古典密码中的置换密码 C++代码实现
时间: 2024-10-09 22:11:41 浏览: 57
应用密码学是一门研究如何使用数学原理来保护信息安全的学科,它涉及加密、解密、数据完整性验证以及身份认证等多种技术。其中,置换密码(Caesar Cipher)是一种最古老的加密方法之一,它通过简单的字母替换规则来隐藏信息。
在古典密码学中的置换密码,比如凯撒密码(Caesar Cipher),最常见的做法是将字母表中的每个字符按照固定的位移量向后移动。例如,凯撒密码的基本思想是将字母表中的每一个字母向前移动固定数位,如移动3位,A就变成D,B变E,以此类推。
下面是一个简单的C++代码实现凯撒密码的加密过程:
```cpp
#include <iostream>
#include <string>
std::string caesar_cipher_encrypt(std::string plaintext, int shift) {
std::string ciphertext = "";
for (char c : plaintext) {
if (isalpha(c)) { // 检查是否为字母
char encrypted_char;
if (islower(c))
encrypted_char = static_cast<char>((c + shift - 'a') % 26 + 'a');
else
encrypted_char = static_cast<char>((c + shift - 'A') % 26 + 'A');
ciphertext += encrypted_char;
} else {
ciphertext += c; // 非字母字符直接保留
}
}
return ciphertext;
}
int main() {
std::string input = "Hello, World!";
int shift = 3;
std::string encrypted = caesar_cipher_encrypt(input, shift);
std::cout << "Original text: " << input << "\n";
std::cout << "Encrypted text: " << encrypted << "\n";
return 0;
}
```
在这个例子中,`caesar_cipher_encrypt`函数接受明文和位移量作为参数,然后对输入字符串中的每个字符进行加密处理。非字母字符保持不变。
阅读全文
相关推荐
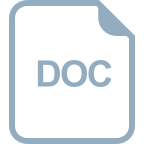
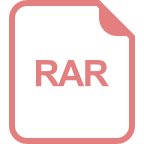















