古典密码学实验(仿射密码)代码编写
时间: 2024-10-14 09:08:21 浏览: 32
古典密码学实验,比如仿射密码(Affine Cipher),是一种基于数学运算的简单密码体制。在Dev-C++环境下,我们可以创建一个小程序来演示这个原理。以下是基本的仿射密码编码和解码步骤的伪代码示例:
```cpp
#include <iostream>
using namespace std;
// 仿射变换函数
char affineTransform(char c, int a, int b) {
const char ALPHABET_UPPER = 'A'; // 大写字母起始位置
const char ALPHABET_LOWER = 'a';
// 处理大写和小写字母
if (c >= 'A' && c <= 'Z') {
int encryptedChar = (a * (c - ALPHABET_UPPER) + b) % 26;
return (encryptedChar + ALPHABET_UPPER);
} else if (c >= 'a' && c <= 'z') {
int encryptedChar = (a * (c - ALPHABET_LOWER) + b) % 26;
return (encryptedChar + ALPHABET_LOWER);
}
return c; // 非字母字符保持不变
}
void encode(const string &plaintext, int a, int b, string &ciphertext) {
for (char c : plaintext) {
ciphertext += affineTransform(c, a, b);
}
}
void decode(const string &ciphertext, int a_inv, int b_inv, string &decrypted) {
for (char c : ciphertext) {
decrypted += affineTransform(c, a_inv, b_inv);
}
}
int main() {
string plaintext = "SECRET MESSAGE";
int key_a = 7, key_b = 3; // 密钥,a和b的值
string ciphertext, decrypted;
// 编码
encode(plaintext, key_a, key_b, ciphertext);
cout << "Original: " << plaintext << endl;
cout << "Encoded: " << ciphertext << endl;
// 解码
int a_inv = extendedEuclidean(key_a, 26); // 计算a的逆元用于解密
decode(ciphertext, a_inv, -(a_inv * key_b) % 26, decrypted);
cout << "Decoded: " << decrypted << endl;
return 0;
}
// 扩展欧几里得算法用于求解模反元素(这里为了简化,假设26是质数)
int extendedEuclidean(int a, int m) {
// ... 算法实现略 ...
}
```
这个程序首先定义了仿射变换函数,然后分别实现了编码和解码的功能。注意在解码时需要先计算出`a`对于模26的逆元。
阅读全文
相关推荐
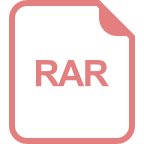
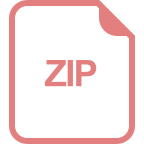
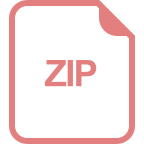















