C++、easyx五子棋
时间: 2024-06-15 09:06:05 浏览: 167
C++是一种通用的编程语言,它支持面向对象的编程范式。C++语言具有高效性和灵活性,广泛应用于系统开发、游戏开发、嵌入式系统等领域。
EasyX是一个简单易用的图形库,它基于C++语言开发,可以方便地进行图形界面的设计和开发。EasyX提供了丰富的绘图函数和图形控件,使得开发者可以快速实现各种图形界面应用。
五子棋是一种古老的棋类游戏,它的目标是在一个棋盘上先将自己的五个棋子连成一条线的玩家获胜。在C++中使用EasyX库可以很方便地实现五子棋游戏的图形界面。
相关问题
C++easyx 五子棋游戏人机对战,人人对战,具有悔棋,复盘等操作。怎么通过控制台和鼠标实现流程
要通过控制台和鼠标实现五子棋游戏的流程,需要使用C++easyx图形库和相关的鼠标事件处理函数。具体实现步骤如下:
1. 初始化游戏界面,并绘制出棋盘和棋子。
2. 判断游戏模式,人机对战或人人对战。
3. 使用鼠标事件处理函数,监听玩家的鼠标操作,并根据鼠标点击的位置落子。
4. 实现悔棋和复盘功能,当玩家点击悔棋按钮时,撤销上一次落子的操作,并将棋盘状态回退到上一个状态;当玩家点击复盘按钮时,显示出之前的棋局状态。
5. 实现胜负判断,当某一方获胜时,弹出胜利提示框。
6. 实现重置游戏功能,当玩家点击重置按钮时,清空棋盘并重新开始游戏。
在实现这些功能时,需要使用到一些C++easyx图形库中的函数,如绘制线条、矩形、圆形等函数,还需要用到鼠标事件处理函数,如`GetMouseMsg`、`MouseHit`等函数。同时,需要使用一些算法来判断胜负,如判断是否连成五子、判断某一方是否占满整个棋盘等。
c++用easyX五子棋人机对战代码
以下是一个简单的C++ EasyX五子棋人机对战代码,使用了基本的minimax算法:
```c++
#include <graphics.h>
#include <conio.h>
#include <iostream>
#include <ctime>
using namespace std;
const int ROWS = 15;
const int COLS = 15;
const int BLOCK_SIZE = 40;
const int CHESS_RADIUS = 15;
int chessBoard[ROWS][COLS] = {0}; // 0表示空,1表示玩家,-1表示电脑
void init();
void drawChessBoard();
void playerMove();
void computerMove();
int evaluate();
int minimax(int depth, int alpha, int beta, bool isMaxPlayer);
int main()
{
init();
while (true)
{
playerMove();
if (evaluate() == 10000)
{
cout << "You win!" << endl;
break;
}
computerMove();
if (evaluate() == -10000)
{
cout << "You lose!" << endl;
break;
}
}
getch();
closegraph();
return 0;
}
void init()
{
initgraph(COLS * BLOCK_SIZE, ROWS * BLOCK_SIZE);
drawChessBoard();
srand(time(NULL));
}
void drawChessBoard()
{
setbkcolor(WHITE);
cleardevice();
setlinecolor(BLACK);
for (int i = 1; i < ROWS; i++)
{
line(BLOCK_SIZE, i * BLOCK_SIZE, COLS * BLOCK_SIZE - BLOCK_SIZE, i * BLOCK_SIZE);
}
for (int i = 1; i < COLS; i++)
{
line(i * BLOCK_SIZE, BLOCK_SIZE, i * BLOCK_SIZE, ROWS * BLOCK_SIZE - BLOCK_SIZE);
}
}
void playerMove()
{
int x, y;
while (true)
{
cout << "Enter the row and column you want to place your chess: ";
cin >> x >> y;
if (x >= 1 && x <= ROWS && y >= 1 && y <= COLS && chessBoard[x - 1][y - 1] == 0)
{
break;
}
else
{
cout << "Invalid move, please try again." << endl;
}
}
chessBoard[x - 1][y - 1] = 1;
setfillcolor(BLACK);
solidcircle(y * BLOCK_SIZE, x * BLOCK_SIZE, CHESS_RADIUS);
}
void computerMove()
{
int bestScore = INT_MIN;
int bestRow = -1;
int bestCol = -1;
for (int i = 0; i < ROWS; i++)
{
for (int j = 0; j < COLS; j++)
{
if (chessBoard[i][j] == 0)
{
chessBoard[i][j] = -1;
int score = minimax(0, INT_MIN, INT_MAX, false);
chessBoard[i][j] = 0;
if (score > bestScore)
{
bestScore = score;
bestRow = i;
bestCol = j;
}
}
}
}
chessBoard[bestRow][bestCol] = -1;
setfillcolor(RED);
solidcircle((bestCol + 1) * BLOCK_SIZE, (bestRow + 1) * BLOCK_SIZE, CHESS_RADIUS);
}
int evaluate()
{
// 检查行
for (int i = 0; i < ROWS; i++)
{
int sum = 0;
for (int j = 0; j < COLS; j++)
{
sum += chessBoard[i][j];
}
if (sum == 5)
{
return 10000;
}
else if (sum == -5)
{
return -10000;
}
}
// 检查列
for (int j = 0; j < COLS; j++)
{
int sum = 0;
for (int i = 0; i < ROWS; i++)
{
sum += chessBoard[i][j];
}
if (sum == 5)
{
return 10000;
}
else if (sum == -5)
{
return -10000;
}
}
// 检查对角线
int sum1 = 0, sum2 = 0;
for (int i = 0; i < ROWS; i++)
{
sum1 += chessBoard[i][i];
sum2 += chessBoard[i][COLS - 1 - i];
}
if (sum1 == 5 || sum2 == 5)
{
return 10000;
}
else if (sum1 == -5 || sum2 == -5)
{
return -10000;
}
return 0;
}
int minimax(int depth, int alpha, int beta, bool isMaxPlayer)
{
int score = evaluate();
if (score != 0)
{
return score;
}
if (depth == 3)
{
return 0;
}
if (isMaxPlayer)
{
int bestScore = INT_MIN;
for (int i = 0; i < ROWS; i++)
{
for (int j = 0; j < COLS; j++)
{
if (chessBoard[i][j] == 0)
{
chessBoard[i][j] = -1;
int score = minimax(depth + 1, alpha, beta, false);
chessBoard[i][j] = 0;
bestScore = max(bestScore, score);
alpha = max(alpha, bestScore);
if (beta <= alpha)
{
return bestScore;
}
}
}
}
return bestScore;
}
else
{
int bestScore = INT_MAX;
for (int i = 0; i < ROWS; i++)
{
for (int j = 0; j < COLS; j++)
{
if (chessBoard[i][j] == 0)
{
chessBoard[i][j] = 1;
int score = minimax(depth + 1, alpha, beta, true);
chessBoard[i][j] = 0;
bestScore = min(bestScore, score);
beta = min(beta, bestScore);
if (beta <= alpha)
{
return bestScore;
}
}
}
}
return bestScore;
}
}
```
注意:该代码只是一个简单的实现,并没有考虑很多复杂的情况,可以根据自己的需求进行修改和优化。
阅读全文
相关推荐
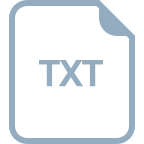
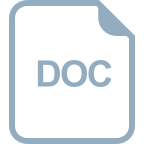




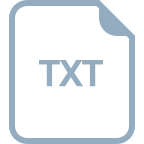
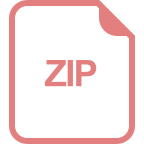
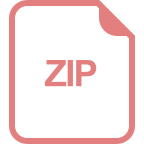
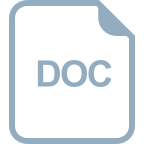
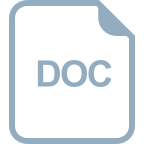
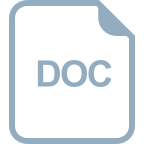