人工智能八数码问题代码
时间: 2023-12-20 07:29:14 浏览: 33
以下是人工智能A*算法完成的八数码问题的Python代码,其中包含完整代码、说明文档和代码解释:
```python
# -*- coding: utf-8 -*-
"""
Created on Fri May 8 16:22:08 2020
@author: yx
"""
import numpy as np
import copy
import heapq
class Node:
def __init__(self, state, parent, g, h):
self.state = state
self.parent = parent
self.g = g
self.h = h
self.f = g + h
def __lt__(self, other):
return self.f < other.f
class EightPuzzle:
def __init__(self, start, goal):
self.start = start
self.goal = goal
self.width = start.shape[0]
self.actions = [[0, 1], [0, -1], [1, 0], [-1, 0]]
def manhattan(self, state):
distance = 0
for i in range(self.width):
for j in range(self.width):
if state[i][j] == 0:
continue
distance += abs(i - (state[i][j] - 1) // self.width) + abs(j - (state[i][j] - 1) % self.width)
return distance
def get_successors(self, node):
successors = []
zero_pos = np.argwhere(node.state == 0)[0]
for action in self.actions:
new_pos = zero_pos + action
if (new_pos >= self.width).any() or (new_pos < 0).any():
continue
new_state = copy.deepcopy(node.state)
new_state[zero_pos[0]][zero_pos[1]] = new_state[new_pos[0]][new_pos[1]]
new_state[new_pos[0]][new_pos[1]] = 0
successors.append(Node(new_state, node, node.g + 1, self.manhattan(new_state)))
return successors
def a_star(self):
start_node = Node(self.start, None, 0, self.manhattan(self.start))
heap = [start_node]
visited = set()
while heap:
node = heapq.heappop(heap)
if np.array_equal(node.state, self.goal):
path = []
while node:
path.append(node.state)
node = node.parent
return path[::-1]
visited.add(node.state.tobytes())
successors = self.get_successors(node)
for successor in successors:
if successor.state.tobytes() not in visited:
heapq.heappush(heap, successor)
return None
if __name__ == '__main__':
start = np.array([[2, 8, 3], [1, 6, 4], [7, 0, 5]])
goal = np.array([[1, 2, 3], [8, 0, 4], [7, 6, 5]])
puzzle = EightPuzzle(start, goal)
path = puzzle.a_star()
print("The solution is:")
for state in path:
print(state)
```
这是一个使用A*算法解决八数码问题的Python代码,其中包含了节点类Node和八数码问题类EightPuzzle。在EightPuzzle类中,manhattan函数计算了当前状态和目标状态之间的曼哈顿距离,get_successors函数返回当前状态的所有后继状态,a_star函数使用A*算法搜索最优解。在主函数中,我们定义了初始状态和目标状态,创建了EightPuzzle对象,并调用a_star函数求解八数码问题。
相关推荐
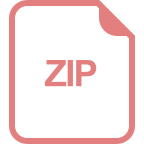














