4.1 数组基本操作 定义两个 3*3的数组,一个是整型数组,一个是 double 型,输入这两个数组的每一个元素的值,找到这两个组的最大值,最小值及其所在的位置。如果有几个值同时最大或者最小,也请输入它们的重复次教并把它们的位置都输出。用c语言编程
时间: 2024-12-19 22:28:37 浏览: 10
在C语言中,我们可以使用嵌套循环来遍历数组,并通过相应的数据结构如`int`和`double`类型的变量分别存储最大值、最小值以及它们出现的次数。以下是完成这个任务的基本步骤:
1. 定义整型和双精度浮点数数组:
```c
int intArray[3][3];
double doubleArray[3][3];
```
2. 输入数组元素:
```c
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
printf("Enter element (%d, %d) of integer array: ", i, j);
scanf("%d", &intArray[i][j]);
printf("Enter element (%d, %d) of double array: ", i, j);
scanf("%lf", &doubleArray[i][j]);
}
}
```
3. 找到最大值、最小值及其位置:
```c
int maxInt = intArray[0][0], minInt = intArray[0][0], maxCount = 1;
int maxPos[2] = {0, 0}, minPos[2] = {0, 0};
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (intArray[i][j] > maxInt) {
maxInt = intArray[i][j];
maxPos[0] = i;
maxPos[1] = j;
maxCount = 1;
} else if (intArray[i][j] == maxInt) {
maxCount++;
}
if (intArray[i][j] < minInt) {
minInt = intArray[i][j];
minPos[0] = i;
minPos[1] = j;
}
}
}
double maxDouble = doubleArray[0][0], minDouble = doubleArray[0][0];
```
4. 对于double数组,同样遍历并更新最大值、最小值及位置,这里省略细节。
5. 输出结果:
```c
printf("Max value in integer array: %d at position (%d,%d), repeated %d times.\n", maxInt, maxPos[0], maxPos[1], maxCount);
printf("Min value in integer array: %d at position (%d,%d).\n", minInt, minPos[0], minPos[1]);
printf("Max value in double array: %lf at position (%d,%d).\n", maxDouble, ...); // 更新此处的索引
printf("Min value in double array: %lf at position (%d,%d).\n", minDouble, ...); // 更新此处的索引
```
阅读全文
相关推荐
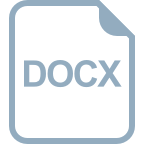
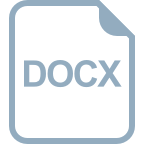
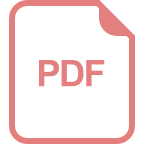
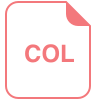
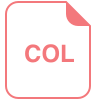
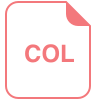
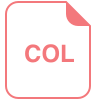
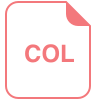
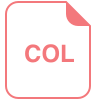
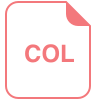
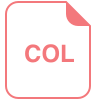
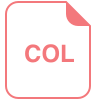
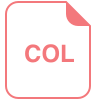
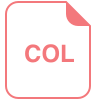
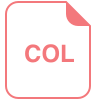
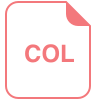
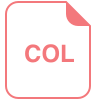
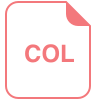