装载问题分支限界法c++
时间: 2024-06-22 19:00:26 浏览: 142
装载问题分支限界法(Branch and Bound)是一种用于求解组合优化问题的算法,特别适用于那些具有大量可能解和部分有序结构的搜索空间。在C++中实现装载问题分支限界法,比如旅行商问题(Traveling Salesman Problem, TSP)或车辆路径问题(Vehicle Routing Problem, VRP),通常会涉及以下步骤:
1. **定义问题**:明确装载物品的限制(如车辆容量、重量、体积等)、物品的起始位置以及目标是找到最短路径或最小成本。
2. **状态表示**:使用数组、vector或类似数据结构来表示当前装载状态,记录每个位置的装载情况。
3. **搜索树结构**:创建一个搜索树,根节点代表初始状态,子节点由可能的选择(比如选择一个未装载的物品或改变一个已装载物品的位置)产生。
4. **分支策略**:从每个节点生成一组可行的子节点,通常通过枚举可能的动作来实现。
5. **评估函数**:为每个节点计算一个估价函数,这个函数估计当前状态到最优解的代价,常见的有启发式函数(如欧几里得距离的总和)。
6. **剪枝策略**:利用上界(upper bound)和下界(lower bound)比较,如果发现某个分支不可能优于当前最优解,就提前结束搜索,避免无效探索。
7. **递归调用**:对每个子节点递归地应用分支限界法,直到达到叶子节点或达到预定的停止条件。
8. **回溯**:当找到最优解时,回溯搜索树以获取完整的解决方案路径。
相关问题
分支限界法处理装载问题,直接给c++代码
好的,以下是使用分支限界法处理装载问题的C++代码:
```c++
#include <iostream>
#include <queue>
#include <algorithm>
using namespace std;
const int MAXN = 1005;
int c, n, w[MAXN];
bool vis[MAXN];
struct Node {
int id;
int rest;
int cost;
bool operator < (const Node &rhs) const {
return cost > rhs.cost;
}
};
int dfs(int id, int rest) {
if(rest <= 0) return 0;
if(id == n + 1) return 0;
if(w[id] > rest) return dfs(id + 1, rest);
return max(dfs(id + 1, rest), dfs(id + 1, rest - w[id]) + 1);
}
int bfs() {
priority_queue<Node> q;
for(int i = 1; i <= n; i++) {
q.push({i, c - w[i], w[i]});
}
while(!q.empty()) {
Node cur = q.top();
q.pop();
if(cur.rest < 0) continue;
if(cur.id == n) return cur.cost;
vis[cur.id] = true;
q.push({cur.id + 1, cur.rest, cur.cost});
q.push({cur.id + 1, cur.rest - w[cur.id + 1], cur.cost + 1});
}
return 0;
}
int main() {
cin >> c >> n;
for(int i = 1; i <= n; i++) {
cin >> w[i];
}
int ans = dfs(1, c); // dfs 求解
cout << ans << endl;
// bfs 求解
cout << bfs() << endl;
return 0;
}
```
其中,dfs函数是使用深度优先搜索求解的方法,bfs函数则使用分支限界法求解,其中用到了一个优先队列来维护搜索状态。两种方法都能够求解出装载问题的最优解。
用分支限界法处理装载问题,直接给c++代码
以下是使用分支限界法处理装载问题的C++代码:
```c++
#include <iostream>
#include <queue>
#include <algorithm>
#include <cstring>
using namespace std;
// 物品结构体
struct Item {
int w; // 物品重量
int id; // 物品编号
};
// 节点结构体
struct Node {
int level; // 当前节点所在层数
int profit; // 当前节点的价值
int weight; // 当前节点的重量
bool operator<(const Node& rhs) const {
return profit < rhs.profit; // 以价值为优先级
}
};
const int MAXN = 100; // 最大物品数量
const int MAXW = 100; // 最大背包容量
int n, W; // 物品数量、背包容量
Item items[MAXN]; // 物品数组
bool used[MAXN]; // 标记物品是否被选中
// 计算上界(即松弛问题的最优解)
int upper_bound(int level, int weight, int profit) {
int bound = profit;
int w = weight;
for (int i = level; i < n; i++) {
if (w + items[i].w <= W) {
w += items[i].w;
bound += items[i].id;
} else {
int remain = W - w;
bound += items[i].id * (double)remain / items[i].w;
break;
}
}
return bound;
}
// 分支限界法求解装载问题
int knapsack() {
priority_queue<Node> pq; // 优先队列
Node u, v;
u.level = 0;
u.profit = 0;
u.weight = 0;
pq.push(u); // 将根节点加入队列
int maxprofit = 0;
while (!pq.empty()) {
u = pq.top(); pq.pop();
if (u.profit > maxprofit) {
maxprofit = u.profit;
}
if (u.level == n) continue;
// 不选当前物品的子节点
v.level = u.level + 1;
v.weight = u.weight;
v.profit = u.profit;
pq.push(v);
// 选当前物品的子节点
v.weight = u.weight + items[u.level].w;
v.profit = u.profit + items[u.level].id;
v.profit += upper_bound(v.level, v.weight, v.profit); // 加上上界
pq.push(v);
}
return maxprofit;
}
int main() {
cin >> n >> W;
for (int i = 0; i < n; i++) {
cin >> items[i].w;
items[i].id = i + 1;
}
sort(items, items + n, [](const Item& a, const Item& b){ // 按单位重量价值排序
return a.id * b.w > b.id * a.w;
});
memset(used, false, sizeof(used));
cout << knapsack() << endl;
return 0;
}
```
注意,这里的 `upper_bound` 函数计算的是松弛问题的最优解,而不是实际问题的最优解。当然,这个上界也可以通过其他方法来计算,比如线性规划等。
阅读全文
相关推荐
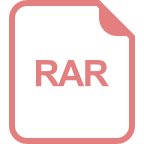
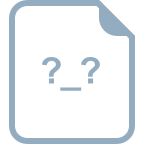
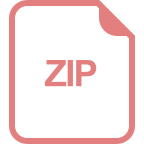



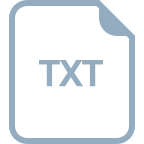
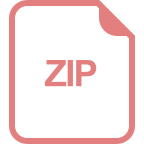
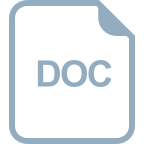
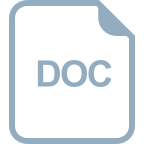

