const auto& element : numbers
时间: 2024-03-07 12:16:40 浏览: 36
`const auto& element` 是一个范围-based for 循环的语法,用于遍历容器中的元素。在这个例子中,`numbers` 可以是一个 QVector 或任何支持范围迭代的容器。
这是一个示例代码:
```cpp
#include <QVector>
#include <QDebug>
int main()
{
QVector<int> numbers = {1, 2, 3, 4, 5};
// 使用范围-based for 循环遍历 numbers 中的元素
for (const auto& element : numbers) {
qDebug() << element;
}
return 0;
}
```
这段代码将输出以下结果:
```
1
2
3
4
5
```
在这个示例中,我们使用范围-based for 循环遍历了 `numbers` 向量中的每个元素。`const auto& element` 定义了一个常量引用 `element`,它将依次引用 `numbers` 中的每个元素。在循环体内部,我们将 `element` 的值输出到控制台。
相关问题
const auto& element : temp
`const auto& element : temp` 是C++中的一个范围-based for循环语法,用于遍历一个序列(如vector、数组等)中的元素。在这个语法中,`element` 是一个临时变量,用于存储每次迭代中的元素值。
`const` 关键字表示 `element` 是一个常量引用,它保证了在循环体内不会修改 `temp` 中的元素。使用引用可以提高性能,避免进行不必要的复制操作。
整个循环的意义是将 `temp` 中的元素依次赋值给 `element`,这样你可以在循环体内使用 `element` 来访问 `temp` 中的每个元素。
例如,在以下代码中:
```cpp
#include <iostream>
#include <vector>
int main() {
std::vector<int> temp = {1, 2, 3, 4, 5};
for (const auto& element : temp) {
std::cout << element << " ";
}
return 0;
}
```
输出结果为:
```
1 2 3 4 5
```
在每次迭代中,`element` 变量分别存储了 `temp` 中的每个元素,并打印出来。
希望这样解释清楚了你的疑问。如果有任何其他问题,请随时提问。
const auto&x:range
`const auto& x` is a syntax in C++ used to define a constant reference to an element in a range. The `range` here can be any iterable container such as an array, vector, or list.
By using `const auto& x`, you are declaring a constant reference (`const`) named `x` that will refer to each element in the `range`. The `auto` keyword allows the compiler to deduce the type of the elements in the range automatically.
Here's an example to illustrate its usage:
```cpp
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& x : numbers) {
std::cout << x << " ";
}
return 0;
}
```
In the above code, the `numbers` vector is iterated using a range-based for loop. The `const auto& x` declares a constant reference `x` that will refer to each element in `numbers`. The `const` qualifier ensures that the elements cannot be modified within the loop body.
相关推荐
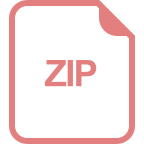
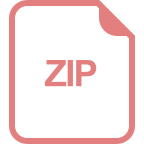
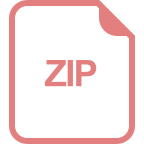












