C++使用RSA算法实现公钥循环解密
时间: 2024-10-17 15:09:23 浏览: 7
在C++中,RSA(Rivest-Shamir-Adleman)算法是一种常用的非对称加密技术,用于加密和解密数据。公钥加密系统的核心是两个密钥对:公钥(public key)和私钥(private key)。公钥可以公开分发给任何人,用于加密信息,而只有拥有对应私钥的人才能解密。
要实现公钥循环解密,通常涉及以下几个步骤:
1. **生成密钥对**:使用RSA库(如 OpenSSL 或者 C++自带的 Crypto++ 等)生成一对密钥,包括公钥(e、n)和私钥(d、p、q),其中e是公钥指数,n是模数,d是私钥逆元,p和q是质因子。
2. **加密过程**:发送方使用接收方的公钥(e和n)将明文转换成密文。这通常是通过将明文表示为整数,然后进行模指数运算(c = m^e mod n)完成。
3. **解密过程**:接收方使用自己的私钥(d、p、q)进行解密,即密文进行解模指数运算(m = c^d mod n),这里的d是私钥的逆元,使得(m^e)^d ≡ m^(ed) mod n 能够还原出原始明文。
4. **循环解密**:如果需要多次解密,只需反复使用相同的私钥解密即可,因为RSA加密保证了只要能计算出解密密钥,就可以无限次地解密。
在C++中,这涉及到库函数的调用和数据结构的处理,比如`CryptoPP`库就提供了一些类和操作来支持RSA算法。例如:
```cpp
#include "cryptopp/osrng.h"
#include "cryptopp/modes.h"
#include "cryptopp/publickey.h"
// 使用Crypto++库
AutoSeededRandomPool prng;
RSA::PublicKey publicKey;
RSA::PrivateKey privateKey;
// ...(密钥生成过程)
byte encryptedData[]; // 加密后的数据
size_t encryptedSize;
std::string decryptedData;
// 解密循环
while (encryptedData != nullptr) {
int remainingLength = decryptedData.size();
if (remainingLength > encryptedSize)
remainingLength = encryptedSize;
// 实现解密...
CryptoPP::PKCS1_OAEP_Decryptor decryptor(publicKey, prng);
if (!decryptor.DecryptBlock(encryptedData, &decryptedData[0], remainingLength))
break; // 解密失败
// 处理解密后的数据...
encryptedData += remainingLength;
encryptedSize -= remainingLength;
}
```
阅读全文
相关推荐
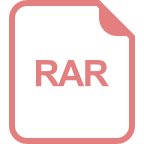
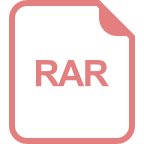
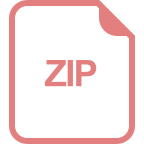















