试编写一个函数,实现在链式存储方式下字符串的replace(S,T1,T2)运算。 试编写一个函数,实现在顺序存储方式 用C++实现以上功能
时间: 2024-12-19 07:21:43 浏览: 10
在C++中,你可以使用`std::string`的内置`replace`函数来替换字符串中的子串,无论是顺序存储还是链式存储。对于链表存储,由于`std::string`默认是以顺序方式存储字符,所以直接使用即可。下面是一个简单的例子:
```cpp
#include <string>
// 对于顺序存储(如std::string)
void replaceSequential(std::string& str, const std::string& oldSubStr, const std::string& newSubStr) {
size_t pos = str.find(oldSubStr);
while (pos != std::string::npos) {
str.replace(pos, oldSubStr.length(), newSubStr);
pos = str.find(oldSubStr, pos + newSubStr.length()); // 继续查找下一个位置
}
}
// 对于链式存储的字符串,这里假设有一个自定义的Node类,每个节点包含一个字符和指向下一个节点的指针
// 由于链表结构复杂,这里不再详细展示如何转换成字符串,一般需要遍历链表并逐个字符构建新的字符串
class Node {
public:
char data;
Node* next;
};
void replaceChain(Node* head, const std::string& oldSubStr, const std::string& newSubStr) {
if (!head || !oldSubStr.empty()) {
Node* current = head;
for (size_t i = 0; i < oldSubStr.length(); ++i) {
while (current && current->data != oldSubStr[i]) {
current = current->next;
}
if (!current) break; // 如果老的子串不存在,则退出
current->data = newSubStr[i];
current = current->next;
}
}
}
```
阅读全文
相关推荐
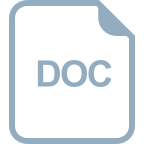
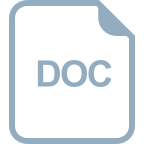
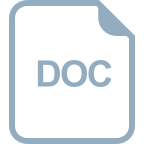












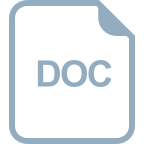
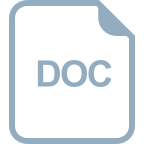
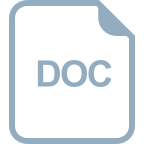
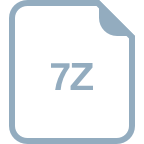
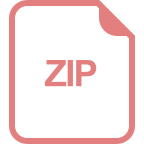