C语言编写一个Shell脚本,实现以下功能: 检查指定目录是否存在,如果不存在则创建该目录。 将当前目录下的所有.txt文件移动到指定目录中。 列出移动后的目录内容。
时间: 2024-10-09 12:10:24 浏览: 48
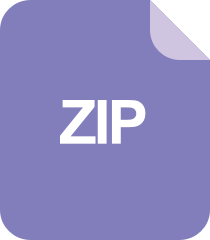
C语言中文网shell脚本教程
在C语言中,我们通常不会直接编写shell脚本,因为C语言主要用于系统编程和底层操作,而shell脚本更常用于操作系统层面的任务自动化。然而,你可以通过调用shell命令来完成这个任务。以下是一个C程序,它包含了实现这些功能所需的步骤:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
#include <sys/stat.h>
#include <unistd.h>
#include <fcntl.h> // 对于rename函数
void check_and_create_dir(const char* dir_path) {
struct stat sb;
if (stat(dir_path, &sb) == -1 && mkdir(dir_path, S_IRWXU | S_IRGRP | S_IROTH) == -1) {
perror("Failed to create directory");
exit(EXIT_FAILURE);
} else if (stat(dir_path, &sb) == -1) {
printf("Directory does not exist, created it.\n");
} else {
printf("Directory exists.\n");
}
}
void move_files_to_dir(const char* src_dir, const char* dest_dir) {
DIR* src_dirp = opendir(src_dir);
if (!src_dirp) {
perror("Failed to open source directory");
return;
}
struct dirent* entry;
while ((entry = readdir(src_dirp))) {
if (strstr(entry->d_name, ".txt")) {
char src_file_path[PATH_MAX] = {0};
strcpy(src_file_path, src_dir);
strcat(src_file_path, "/");
strcat(src_file_path, entry->d_name);
char dest_file_path[PATH_MAX] = {0};
strcpy(dest_file_path, dest_dir);
strcat(dest_file_path, "/");
strcat(dest_file_path, entry->d_name);
if (rename(src_file_path, dest_file_path) == -1) {
perror("Failed to rename file");
continue;
}
printf("Moved '%s' to '%s'\n", src_file_path, dest_file_path);
}
}
closedir(src_dirp);
}
void list_directory_contents(const char* dir_path) {
DIR* dirp = opendir(dir_path);
if (!dirp) {
perror("Failed to open directory");
return;
}
struct dirent* entry;
printf("\nContents of %s:\n", dir_path);
while ((entry = readdir(dirp))) {
printf("%s\n", entry->d_name);
}
closedir(dirp);
}
int main() {
const char* src_dir = "./source"; // 源目录
const char* dest_dir = "./destination"; // 目标目录
check_and_create_dir(dest_dir);
move_files_to_dir(src_dir, dest_dir);
list_directory_contents(dest_dir);
return 0;
}
```
这个C程序首先检查并创建目标目录,然后从源目录移动所有的`.txt`文件到目标目录,并最后列出目标目录的内容。
请注意,这个例子假设了源目录和目标目录都在当前工作目录下。在实际应用中,你需要提供正确的路径。
阅读全文
相关推荐
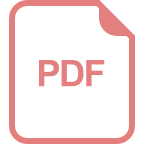
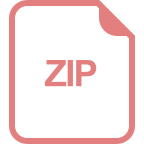
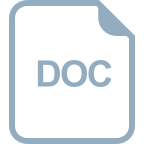
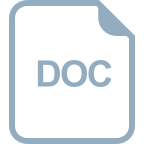
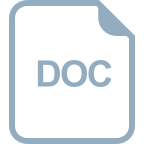
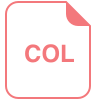





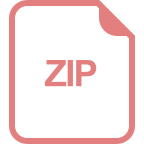
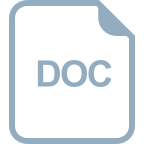



