import os os.makedirs(os.path.join('..','data'),exist_ok=True) data_file = os.path.join('..','data','house_tiny.csv') with open(data_file,'w') as f: f.write('NumRooms,Alley,Price\n')#列名 f.write('NA,Pave,127500\n') #每行表示一个数据样本 f.write('2,NA,106000\n') f.write('4,NA,178100\n') f.write('NA,NA,140000\n')
时间: 2024-01-08 09:02:32 浏览: 26
这段代码的作用是创建一个文件夹和一个名为 'house_tiny.csv' 的数据文件,并将一些数据写入该文件中。
首先,使用 `os.makedirs` 函数创建一个名为 'data' 的文件夹。`os.makedirs` 函数的第一个参数是要创建的文件夹的路径,这里使用 `os.path.join` 函数将路径拼接为 '..'(上级目录)和 'data'。`exist_ok=True` 参数表示如果文件夹已经存在,则不会引发错误。
接下来,定义一个变量 `data_file`,用于保存数据文件的路径。使用 `os.path.join` 函数将路径拼接为 '..'、'data' 和 'house_tiny.csv'。
然后,使用 `open` 函数打开 `data_file` 文件,并以写入模式打开。使用关键字 `with` 语句可以确保文件在使用后被正确关闭。
在文件打开后,代码使用 `f.write` 函数写入数据。首先写入列名,即 'NumRooms,Alley,Price'。然后,每行表示一个数据样本,每个字段用逗号分隔。在这个例子中,有四个数据样本。第一个样本的字段值分别为 'NA'、'Pave' 和 '127500',第二个样本的字段值分别为 '2'、'NA' 和 '106000',以此类推。
最后,文件写入完毕后,会自动关闭文件。
这段代码的目的是创建一个包含一些房屋数据的 CSV 文件。
相关问题
此代码import os import numpy as np from PIL import Image def process_image(image_path, save_path): # 读取nii文件 image_array = np.load(image_path).astype(np.float32) # 归一化到0-255之间 image_array = (image_array - np.min(image_array)) / (np.max(image_array) - np.min(image_array)) * 255 # 将数据类型转换为uint8 image_array = image_array.astype(np.uint8) # 将三维图像分成若干个二维图像 for i in range(image_array.shape[0]): image = Image.fromarray(image_array[i]) image.save(os.path.join(save_path, f"{i}.png")) def process_label(label_path, save_path): # 读取nii文件 label_array = np.load(label_path).astype(np.uint8) # 将标签转换为灰度图 label_array[label_array == 1] = 255 label_array[label_array == 2] = 128 # 将三维标签分成若干个二维标签 for i in range(label_array.shape[0]): label = Image.fromarray(label_array[i]) label.save(os.path.join(save_path, f"{i}.png")) # LiTS2017数据集路径 data_path = "C:\\Users\\Administrator\\Desktop\\LiTS2017" # 保存路径 save_path = "C:\\Users\\Administrator\\Desktop\\2D-LiTS2017" # 创建保存路径 os.makedirs(save_path, exist_ok=True) os.makedirs(os.path.join(save_path, "image"), exist_ok=True) os.makedirs(os.path.join(save_path, "mask"), exist_ok=True) # 处理Training Batch 1 image_path = os.path.join(data_path, "Training Batch 1", "volume-{}.npy") for i in range(131): process_image(image_path.format(i), os.path.join(save_path, "image")) # 处理Training Batch 2 label_path = os.path.join(data_path, "Training Batch 2", "segmentation-{}.npy") for i in range(131): process_label(label_path.format(i), os.path.join(save_path, "mask"))出现FileNotFoundError: [Errno 2] No such file or directory: 'C:\\Users\\Administrator\\Desktop\\LiTS2017\\Training Batch 1\\volume-0.npy',修复它,并给出完整代码
首先,这个错误提示表明程序找不到第一个npy文件。因此,需要检查路径是否正确。
同时,还需要确保所有的路径和文件名都是正确的。下面是修复后的完整代码:
```python
import os
import numpy as np
from PIL import Image
def process_image(image_path, save_path):
# 读取nii文件
image_array = np.load(image_path).astype(np.float32)
# 归一化到0-255之间
image_array = (image_array - np.min(image_array)) / (np.max(image_array) - np.min(image_array)) * 255
# 将数据类型转换为uint8
image_array = image_array.astype(np.uint8)
# 将三维图像分成若干个二维图像
for i in range(image_array.shape[0]):
image = Image.fromarray(image_array[i])
image.save(os.path.join(save_path, f"{i}.png"))
def process_label(label_path, save_path):
# 读取nii文件
label_array = np.load(label_path).astype(np.uint8)
# 将标签转换为灰度图
label_array[label_array == 1] = 255
label_array[label_array == 2] = 128
# 将三维标签分成若干个二维标签
for i in range(label_array.shape[0]):
label = Image.fromarray(label_array[i])
label.save(os.path.join(save_path, f"{i}.png"))
# LiTS2017数据集路径
data_path = "C:\\Users\\Administrator\\Desktop\\LiTS2017"
# 保存路径
save_path = "C:\\Users\\Administrator\\Desktop\\2D-LiTS2017"
# 创建保存路径
os.makedirs(save_path, exist_ok=True)
os.makedirs(os.path.join(save_path, "image"), exist_ok=True)
os.makedirs(os.path.join(save_path, "mask"), exist_ok=True)
# 处理Training Batch 1
image_path = os.path.join(data_path, "Training Batch 1", "volume-{}.npy")
for i in range(131):
if os.path.exists(image_path.format(i)):
process_image(image_path.format(i), os.path.join(save_path, "image"))
else:
print(f"{image_path.format(i)} not exists!")
# 处理Training Batch 2
label_path = os.path.join(data_path, "Training Batch 2", "segmentation-{}.npy")
for i in range(131):
if os.path.exists(label_path.format(i)):
process_label(label_path.format(i), os.path.join(save_path, "mask"))
else:
print(f"{label_path.format(i)} not exists!")
```
在这个修复后的代码中,我们添加了对文件是否存在的检查,并输出了相应的提示信息。现在我们可以运行代码进行处理,同时会得到相应的提示信息帮助我们快速定位错误。
import jittor as jt import jrender as jr jt.flags.use_cuda = 1 # 开启GPU加速 import os import tqdm import numpy as np import imageio import argparse # 获取当前文件所在目录路径和数据目录路径 current_dir = os.path.dirname(os.path.realpath(__file__)) data_dir = os.path.join(current_dir, 'data') def main(): # 创建命令行参数解析器 parser = argparse.ArgumentParser() parser.add_argument('-i', '--filename-input', type=str, default=os.path.join(data_dir, 'obj/spot/spot_triangulated.obj')) parser.add_argument('-o', '--output-dir', type=str, default=os.path.join(data_dir, 'results/output_render')) args = parser.parse_args() # other settings camera_distance = 2.732 elevation = 30 azimuth = 0 # load from Wavefront .obj file mesh = jr.Mesh.from_obj(args.filename_input, load_texture=True, texture_res=5, texture_type='surface', dr_type='softras') # create renderer with SoftRas renderer = jr.Renderer(dr_type='softras') os.makedirs(args.output_dir, exist_ok=True) # draw object from different view loop = tqdm.tqdm(list(range(0, 360, 4))) writer = imageio.get_writer(os.path.join(args.output_dir, 'rotation.gif'), mode='I') imgs = [] from PIL import Image for num, azimuth in enumerate(loop): # rest mesh to initial state mesh.reset_() loop.set_description('Drawing rotation') renderer.transform.set_eyes_from_angles(camera_distance, elevation, azimuth) rgb = renderer.render_mesh(mesh, mode='rgb') image = rgb.numpy()[0].transpose((1, 2, 0)) writer.append_data((255*image).astype(np.uint8)) writer.close() # draw object from different sigma and gamma loop = tqdm.tqdm(list(np.arange(-4, -2, 0.2))) renderer.transform.set_eyes_from_angles(camera_distance, elevation, 45) writer = imageio.get_writer(os.path.join(args.output_dir, 'bluring.gif'), mode='I') for num, gamma_pow in enumerate(loop): # rest mesh to initial state mesh.reset_() renderer.set_gamma(10**gamma_pow) renderer.set_sigma(10**(gamma_pow - 1)) loop.set_description('Drawing blurring') images = renderer.render_mesh(mesh, mode='rgb') image = images.numpy()[0].transpose((1, 2, 0)) # [image_size, image_size, RGB] writer.append_data((255*image).astype(np.uint8)) writer.close() # save to textured obj mesh.reset_() mesh.save_obj(os.path.join(args.output_dir, 'saved_spot.obj')) if __name__ == '__main__': main()在每行代码后添加注释
# 引入所需的库
import jittor as jt
import jrender as jr
jt.flags.use_cuda = 1 # 开启GPU加速
import os
import tqdm
import numpy as np
import imageio
import argparse
# 获取当前文件所在目录路径和数据目录路径
current_dir = os.path.dirname(os.path.realpath(__file__))
data_dir = os.path.join(current_dir, 'data')
def main():
# 创建命令行参数解析器
parser = argparse.ArgumentParser()
parser.add_argument('-i', '--filename-input', type=str,
default=os.path.join(data_dir, 'obj/spot/spot_triangulated.obj')) # 输入文件路径
parser.add_argument('-o', '--output-dir', type=str,
default=os.path.join(data_dir, 'results/output_render')) # 输出文件路径
args = parser.parse_args()
# other settings
camera_distance = 2.732 # 相机距离
elevation = 30 # 抬高角度
azimuth = 0 # 方位角度
# load from Wavefront .obj file
mesh = jr.Mesh.from_obj(args.filename_input, load_texture=True, texture_res=5, texture_type='surface', dr_type='softras') # 从.obj文件载入模型
# create renderer with SoftRas
renderer = jr.Renderer(dr_type='softras') # 创建渲染器
os.makedirs(args.output_dir, exist_ok=True)
# draw object from different view
loop = tqdm.tqdm(list(range(0, 360, 4))) # 视角变换循环
writer = imageio.get_writer(os.path.join(args.output_dir, 'rotation.gif'), mode='I') # 创建gif文件
imgs = []
from PIL import Image
for num, azimuth in enumerate(loop):
# rest mesh to initial state
mesh.reset_() # 重置模型状态
loop.set_description('Drawing rotation')
renderer.transform.set_eyes_from_angles(camera_distance, elevation, azimuth) # 设置相机位置和角度
rgb = renderer.render_mesh(mesh, mode='rgb') # 渲染模型
image = rgb.numpy()[0].transpose((1, 2, 0)) # 转置图片通道
writer.append_data((255*image).astype(np.uint8)) # 写入gif文件
writer.close()
# draw object from different sigma and gamma
loop = tqdm.tqdm(list(np.arange(-4, -2, 0.2))) # 模糊循环
renderer.transform.set_eyes_from_angles(camera_distance, elevation, 45) # 设置相机位置和角度
writer = imageio.get_writer(os.path.join(args.output_dir, 'bluring.gif'), mode='I') # 创建gif文件
for num, gamma_pow in enumerate(loop):
# rest mesh to initial state
mesh.reset_() # 重置模型状态
renderer.set_gamma(10**gamma_pow) # 设置gamma值
renderer.set_sigma(10**(gamma_pow - 1)) # 设置sigma值
loop.set_description('Drawing blurring')
images = renderer.render_mesh(mesh, mode='rgb') # 渲染模型
image = images.numpy()[0].transpose((1, 2, 0)) # [image_size, image_size, RGB]
writer.append_data((255*image).astype(np.uint8)) # 写入gif文件
writer.close()
# save to textured obj
mesh.reset_() # 重置模型状态
mesh.save_obj(os.path.join(args.output_dir, 'saved_spot.obj')) # 保存模型
if __name__ == '__main__':
main()
相关推荐
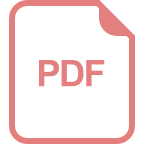
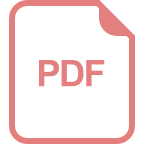
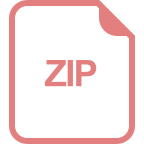












