我想设置一款最简单的贪吃蛇游戏,请你帮我给出代码
时间: 2024-10-19 14:08:17 浏览: 11
当然可以帮你概述一下如何创建一个基本的贪吃蛇游戏。这里我会提供一个使用Python语言的基本框架,它使用了Pygame库来处理图形界面:
```python
import pygame
import sys
# 初始化pygame
pygame.init()
# 设置窗口大小
screen_width = 400
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("贪吃蛇")
snake_color = (0, 255, 0) # 绿色
food_color = (255, 0, 0) # 红色
snake_pos = [(screen_width // 2, screen_height // 2)]
snake_dir = [0, 1] # 向上
# 蛇身体部分的列表
snake_body = [snake_pos]
clock = pygame.time.Clock()
food_spawned = False
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP and snake_dir != [0, -1]:
snake_dir = [-1, 0]
elif event.key == pygame.K_DOWN and snake_dir != [0, 1]:
snake_dir = [1, 0]
elif event.key == pygame.K_LEFT and snake_dir != [1, 0]:
snake_dir = [0, -1]
elif event.key == pygame.K_RIGHT and snake_dir != [-1, 0]:
snake_dir = [0, 1]
snake_pos_new = [snake_pos[0][0] + snake_dir[0], snake_pos[0][1] + snake_dir[1]]
# 检查新位置是否越界或撞到自己
if snake_pos_new[0] < 0 or snake_pos_new[0] >= screen_width or \
snake_pos_new[1] < 0 or snake_pos_new[1] >= screen_height or \
snake_pos_new in snake_body[:-1]:
print("Game Over!")
pygame.quit()
sys.exit()
snake_body.append(snake_pos_new)
snake_body.pop(0)
if not food_spawned:
food_x = random.randint(0, screen_width - 1)
food_y = random.randint(0, screen_height - 1)
while (food_x, food_y) in snake_body:
food_x = random.randint(0, screen_width - 1)
food_y = random.randint(0, screen_height - 1)
food_spawned = True
else:
food_pos = (food_x, food_y)
screen.fill((0, 0, 0)) # 渲染黑色背景
pygame.draw.rect(screen, snake_color, pygame.Rect(snake_pos[0], (10, 10))) # 绘制蛇
pygame.draw.rect(screen, food_color, pygame.Rect(food_pos, (10, 10))) # 绘制食物
pygame.display.update()
clock.tick(10) # 控制帧率
# 结束游戏循环
```
这只是一个基础版的贪吃蛇游戏,它没有复杂的碰撞检测或得分系统。如果你想增加更多功能,比如食物生成、碰撞检测等,你需要继续完善这个代码。
阅读全文
相关推荐
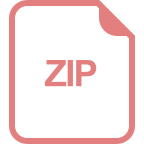
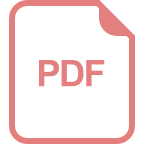
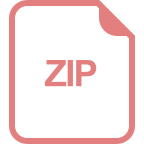
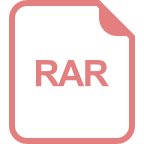
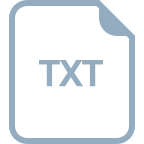
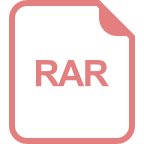
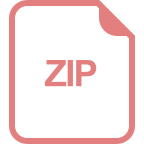
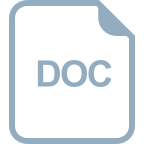









