假设有一个链栈st ,设计一个算法出栈从栈顶开始的第k 个结点
时间: 2024-10-09 16:11:48 浏览: 46
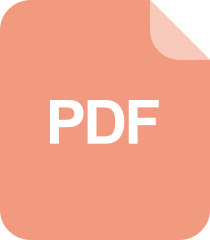
设计一个算法,将一个栈中的元素排序
要在链式栈(Stack)中找到并删除从栈顶开始的第 `k` 个节点,我们可以使用迭代或递归的方式,但因为我们需要同时处理出栈和定位第 `k` 个节点,所以最好选择迭代的方法。以下是一个基于迭代的C++解决方案:
```cpp
#include <iostream>
struct Node {
int data;
Node* next;
};
class StackWithKthNode {
private:
Node* top; // 栈顶指针
int k;
public:
StackWithKthNode() : top(nullptr), k(0) {}
// 入栈操作
void push(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = top;
top = newNode;
++k;
}
// 出栈操作,同时检查是否到达第k个节点
Node* pop_kth_node() {
if (top == nullptr || k <= 0) {
std::cout << "Invalid operation, stack is empty or k is not valid.\n";
return nullptr;
}
if (k == 1) { // 如果k等于1,我们直接弹出栈顶元素
Node* temp = top;
top = top->next;
delete temp;
--k;
return nullptr; // 返回已删除的节点(这里返回nullptr)
} else {
Node* prev = nullptr;
for (int i = 1; i < k && top != nullptr; ++i) {
prev = top;
top = top->next;
}
if (prev != nullptr) { // 找到第k-1个节点,然后继续遍历直到找到第k个
Node* temp = top;
top = top->next;
delete temp;
--k;
}
return nullptr; // 返回的是第k个之后的节点(这里返回nullptr)
}
}
};
int main() {
StackWithKthNode st;
// 添加节点示例
st.push(1);
st.push(2);
st.push(3);
st.push(4);
// 按照需求操作,例如获取第3个节点(从栈顶开始计数)
Node* result = st.pop_kth_node();
if (result) {
std::cout << "The kth node data: " << result->data << "\n"; // 输出结果
delete result; // 释放内存
}
return 0;
}
```
这个程序首先定义了一个链栈结构 `Node` 和一个辅助类 `StackWithKthNode`,包含了入栈、出栈以及查找第 `k` 个节点的方法。请注意,在实际应用中,你需要确保在使用完后正确地清理内存。
阅读全文
相关推荐
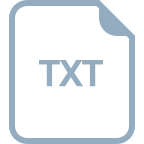
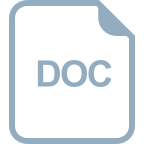















