用java编写一个测试程序,提示用户输入一个n*n的矩阵(n>=6),并输入矩阵中的每个值(自定义)。如果这个矩阵中有四个连续的数字相同,输出true,否则输出false。
时间: 2024-09-13 09:18:24 浏览: 69
要编写这样一个Java测试程序,首先需要创建一个能够接受用户输入的矩阵,并且包含逻辑来判断是否存在四个连续相同的数字。程序的大致步骤如下:
1. 提示用户输入矩阵的大小n,确保n>=6。
2. 根据n的值创建一个n*n的二维数组,并接受用户输入填充该矩阵。
3. 实现逻辑判断矩阵中是否存在连续四个相同的数字。
下面是一个简单的实现示例:
```java
import java.util.Scanner;
public class MatrixFourInARowChecker {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入矩阵大小n(n>=6):");
int n = scanner.nextInt();
if (n < 6) {
System.out.println("矩阵大小不满足要求,请重新运行程序。");
return;
}
int[][] matrix = new int[n][n];
System.out.println("请输入矩阵中的每个值(共 " + n * n + " 个值):");
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
matrix[i][j] = scanner.nextInt();
}
}
boolean result = checkFourInARow(matrix);
System.out.println(result ? "存在四个连续的数字相同" : "不存在四个连续的数字相同");
scanner.close();
}
private static boolean checkFourInARow(int[][] matrix) {
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
// 检查横向、纵向、两个对角线方向
if (checkDirection(matrix, i, j, 1, 0) || // 横向
checkDirection(matrix, i, j, 0, 1) || // 纵向
checkDirection(matrix, i, j, 1, 1) || // 对角线
checkDirection(matrix, i, j, 1, -1)) { // 反对角线
return true;
}
}
}
return false;
}
private static boolean checkDirection(int[][] matrix, int row, int col, int dRow, int dCol) {
int count = 1;
int value = matrix[row][col];
int r, c;
// 向一个方向检查
r = row + dRow;
c = col + dCol;
while (r >= 0 && r < matrix.length && c >= 0 && c < matrix.length && matrix[r][c] == value) {
count++;
r += dRow;
c += dCol;
}
// 向相反方向检查
r = row - dRow;
c = col - dCol;
while (r >= 0 && r < matrix.length && c >= 0 && c < matrix.length && matrix[r][c] == value) {
count++;
r -= dRow;
c -= dCol;
}
return count >= 4;
}
}
```
以上程序会首先检查用户输入的n是否满足最小条件,然后根据用户输入构建矩阵,并调用`checkFourInARow`方法来判断矩阵中是否存在四个连续的相同数字。
阅读全文
相关推荐
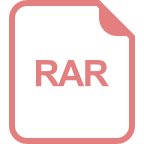
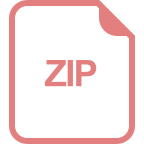
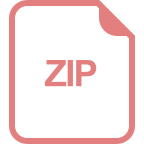





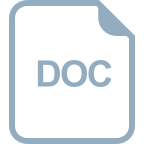









