用c++编写链栈来初始化栈,取出栈顶元素,出栈,入栈。
时间: 2024-10-13 19:05:46 浏览: 53
在 C++ 中,我们可以使用链表数据结构来实现一个链式栈(Linked Stack)。链栈是一种动态数据结构,因为它不需要预先确定存储元素的数量。下面是一个简单的链栈实现:
```cpp
#include <iostream>
// 链栈节点定义
struct Node {
int data;
Node* next;
};
// 初始化空栈
class LinkedStack {
private:
Node* top;
public:
// 构造函数
LinkedStack() : top(nullptr) {}
// 入栈操作(push)
void push(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = top;
top = newNode;
std::cout << "元素 " << value << " 入栈成功\n";
}
// 出栈操作(pop),如果栈为空则返回 -1
int pop() {
if (top == nullptr) {
std::cout << "栈为空,无法出栈\n";
return -1;
}
int poppedValue = top->data;
Node* temp = top;
top = top->next;
delete temp;
std::cout << "元素 " << poppedValue << " 出栈成功\n";
return poppedValue;
}
// 获取栈顶元素,但不删除它
int peek() const {
if (top == nullptr) {
std::cout << "栈为空,无栈顶元素\n";
return -1;
}
return top->data;
}
};
int main() {
LinkedStack stack;
stack.push(10);
stack.push(20);
int topElement = stack.peek(); // 获取栈顶元素:20
std::cout << "当前栈顶元素:" << topElement << "\n";
stack.pop(); // 出栈元素:20
int poppedElement = stack.pop(); // 再次尝试出栈,得到并打印:10
return 0;
}
```
阅读全文
相关推荐
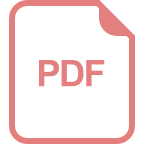
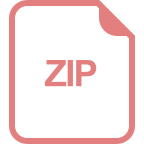


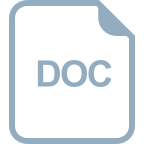












