#include<iostream> #include<algorithm> using namespace std; int cnt = 1; bool col[10]; bool du[20]; bool backdu[40]; int g[10][10]; void dfs(int step){ if(step==9){ printf("No. %d\n",cnt); for(int i=1;i<=8;i++){ for(int j=1;j<=8;j++){ printf("%d ",g[i][j]); } printf("\n"); } ++cnt; } for(int i=1;i<=8;i++){ if(col[i]==false&&du[step+i]==false&&backdu[step-i+20]==false){ col[i] = true; du[step+i]=true; backdu[step-i+20]=true ; g[step][i] = 1; dfs(step+1); col[i] = false; du[step+i]=false; backdu[step-i+20]=false; g[step][i] = 0; } } } int main(){ dfs(1); return 0; }
时间: 2024-04-13 22:27:39 浏览: 96
这是一个经典的八皇后问题的解法,通过深度优先搜索来找到所有的解。代码中使用了三个布尔数组来记录每行、每列、每条对角线上是否已经放置了皇后,然后通过递归调用dfs函数来尝试放置皇后。当放置完八个皇后之后,就找到了一个解,并将解输出。
值得注意的是,这段代码并没有使用C++的iostream库中的printf函数,而是直接使用了stdio.h中的printf函数。此外,代码中没有使用algorithm库。
如果你有任何问题,可以继续问我。
相关问题
#include<iostream> #include<cstring> #include<vector> #include<unordered_map> #include<algorithm> #include<queue> #include<iomanip> #include<cmath> #include <fstream> #include <cstdlib> #include <map> // #define int long long using namespace std; const int N = 200010; int n,m,t,p; int ar[N],s[N],cnt,sum,ans; int dx[4]={0,0,1,-1},dy[4]={1,-1,0,0}; double a,b; string st; struct pe { double num,p; }pv[N]; bool cmp (pe a,pe b) { if(a.p==b.p)return a.num<b.num; return a.p>b.p; } void solve () { cin>>n; for(int i=0;i<n;i++) { cin>>a>>b; pv[i].p=a/(a+b); pv[i].num=i+1; } sort(pv,pv+n,cmp); for(int i=0;i<n;i++)cout<<pv[i].num<<" "; } int main() { ios::sync_with_stdio(0); cin.tie(0); cout.tie(0); solve(); return 0; } 请找出代码错误
代码中没有明显的错误。但是,可能存在一些潜在的问题。
1. 在排序函数`cmp`中,当两个`pe`结构体的`p`值相等时,比较的是`num`值。这可能会导致不稳定的排序结果,如果对结果的顺序有要求,可能需要修改排序函数。
2. `pv`数组的大小为`N`,如果`n`的值大于`N`,可能会导致数组越界。
3. 在读取输入时,没有进行输入合法性检查。如果输入的数据格式不正确,可能会导致程序出错。
4. 程序中定义了一些未使用的变量,如`t`、`m`、`s`、`ar`等。可以考虑删除这些无用的变量。
请注意,以上问题只是可能存在的潜在问题,具体还需要根据实际运行情况来确定错误的原因。
#include <cstdio> #include <iostream> #include <cstring> #include <algorithm> #include <vector> #define maxn 11111 using namespace std; vector<int> a[maxn]; int x,y; int n,m,s; int ans[maxn]; int cnt=1,res=1; bool visited[maxn]; int dfs(int x) { if(visited[x]) return 0; ans[cnt]=x; cnt++; visited[x]=true; for (auto to:a[x]) { if (dfs(to)) { ans[cnt]=x; cnt++; } } return 1; } int main() { cin>>n>>m>>s; for(int i=1;i<=m;i++) { cin>>x>>y; a[x].push_back(y); a[y].push_back(x); } for(int i=1;i<=n;i++) sort(a[i].begin(),a[i].end()); dfs(s); for(int i=1;i<=n;i++) { if(visited[i]==0) { res=0; break; } } //cout<<"res="<<res<<endl; for(int i=1;i<cnt;i++) cout<<ans[i]<<" "; if(res==0) cout<<0<<endl; return 0; }
这段代码是一个深度优先搜索(DFS)遍历无向图的程序,它可以输出从起点s出发的所有路径。其中:
- 输入n代表节点数,m代表边数,s代表起点编号;
- 使用vector数组a来表示图的邻接表,a[i]存储节点i的所有相邻节点;
- 使用visited数组记录每个节点是否被访问过;
- 使用dfs函数进行深度优先搜索,从起点s开始,对于当前节点x,遍历x的每个相邻节点to;
- 如果to未被访问过,就递归调用dfs(to)进行搜索;
- 如果to已被访问过,说明发现了一个环,将环上经过的节点输出,并返回1,表示找到了环;
- 在返回时,将当前节点x加入答案数组ans,并将cnt加1,表示已经访问了一个新节点;
- 最后检查visited数组是否所有位置都为1,如果有未被访问过的节点,则说明图不是连通的,输出0表示不是连通图;
- 输出答案数组ans中存储的所有节点编号,即为从起点s出发的所有路径。
需要注意的是,这段代码使用了vector和sort,可以通过这种方式实现邻接表的排序,从而保证输出的路径是字典序最小的。
阅读全文
相关推荐
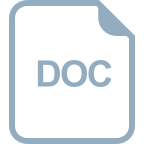
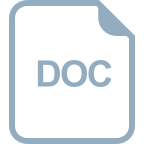
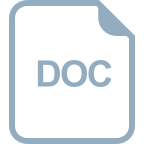














