用C++写一个复数运算
时间: 2024-12-06 14:21:42 浏览: 4
在C++中,我们可以使用结构体或者类来表示复数,并实现加、减、乘、除等基本运算。这里是一个简单的复数结构体和一些基本操作的示例:
```cpp
#include <iostream>
using namespace std;
// 定义复数类 Complex
struct Complex {
double real; // 实部
double imag; // 虚部
// 构造函数
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 运算符重载
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
Complex operator*(const Complex& other) const {
return Complex(real * other.real - imag * other.imag,
real * other.imag + imag * other.real);
}
// 如果除数非零,可以添加一个operator/实现除法
Complex operator/(const Complex& other) const {
if (other.real != 0 && other.imag != 0) {
double denominator = other.real * other.real + other.imag * other.imag;
return Complex((real * other.real + imag * other.imag) / denominator,
(imag * other.real - real * other.imag) / denominator);
}
else {
throw runtime_error("Division by zero is not allowed.");
}
}
// 打印复数
void print() const {
cout << real << " + " << imag << "i" << endl;
}
};
int main() {
Complex c1(3, 4);
Complex c2(1, 2);
Complex sum = c1 + c2;
Complex diff = c1 - c2;
Complex product = c1 * c2;
Complex quotient; // 注意此处没有计算除法,因为没有实现
sum.print(); // 输出结果
diff.print();
return 0;
}
```
在这个例子中,我们通过重载运算符实现了复数的加、减和乘法。`main`函数中展示了如何创建复数对象并进行这些运算。
阅读全文
相关推荐
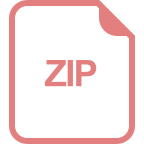
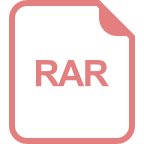
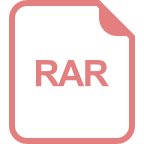
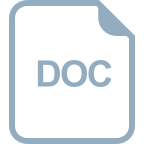
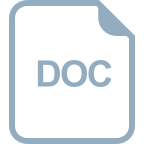
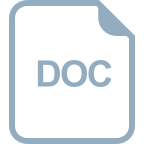
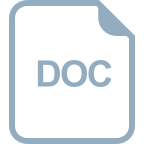
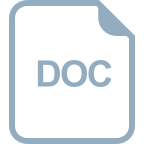






