C++如何处理复数运算?
时间: 2024-11-09 12:29:39 浏览: 8
在C++中,处理复数运算主要是通过标准模板库(Standard Template Library, STL)中的`<complex>`头文件。这个库提供了一种称为`complex`的类,可以用来表示复数以及进行相关的算术运算。
以下是一个简单的例子,展示了如何创建`complex`对象并进行基本的复数运算:
```cpp
#include <iostream>
#include <complex>
int main() {
std::complex<double> c1(1.0, 2.0); // 创建一个复数,实部1.0,虚部2.0
std::complex<double> c2(3.0, 4.0);
// 操作
std::complex<double> sum = c1 + c2; // 加法
std::cout << "Sum: " << sum.real() << "+" << sum.imag() << "i\n"; // 输出复数
std::complex<double> product = c1 * c2; // 乘法
std::cout << "Product: " << product.real() << "+" << product.imag() << "i\n";
return 0;
}
```
在这个例子中,`real()`和`imag()`分别是获取复数的实部和虚部的方法,而`+`和`*`则是对应于复数的加法和乘法运算符。
相关问题
C++语句复数运算程序
下面是一个简单的 C++ 代码示例,用于计算两个复数的加法、减法和乘法运算。
```cpp
#include <iostream>
using namespace std;
struct Complex {
double real, imag;
};
Complex add(Complex a, Complex b) {
Complex res;
res.real = a.real + b.real;
res.imag = a.imag + b.imag;
return res;
}
Complex sub(Complex a, Complex b) {
Complex res;
res.real = a.real - b.real;
res.imag = a.imag - b.imag;
return res;
}
Complex mul(Complex a, Complex b) {
Complex res;
res.real = a.real * b.real - a.imag * b.imag;
res.imag = a.real * b.imag + a.imag * b.real;
return res;
}
int main() {
Complex a, b, res;
char op;
cout << "Enter the first complex number: ";
cin >> a.real >> a.imag;
cout << "Enter the second complex number: ";
cin >> b.real >> b.imag;
cout << "Enter the operation (+, -, *): ";
cin >> op;
switch (op) {
case '+':
res = add(a, b);
cout << "The result of addition is: " << res.real << " + " << res.imag << "i" << endl;
break;
case '-':
res = sub(a, b);
cout << "The result of subtraction is: " << res.real << " + " << res.imag << "i" << endl;
break;
case '*':
res = mul(a, b);
cout << "The result of multiplication is: " << res.real << " + " << res.imag << "i" << endl;
break;
default:
cout << "Invalid operation." << endl;
break;
}
return 0;
}
```
在此代码中,我们定义了一个 `Complex` 结构体来表示复数。它包含实部和虚部两个成员变量。然后,我们定义了三个函数来执行复数的加法、减法和乘法运算。每个函数都接受两个 `Complex` 类型的参数,并返回一个 `Complex` 类型的结果。最后,我们在 `main()` 函数中读入两个复数和一个运算符,并根据运算符调用相应的函数来计算结果。
C++语句复数运算程序需用到重载运算
是的,C++语言支持运算符重载,可以使用运算符来实现复数运算。在进行复数运算时,需要对加、减、乘、除等运算符进行重载。运算符重载可以通过类的成员函数或者全局函数来实现。下面是一个使用类的成员函数实现复数加法的示例代码:
```c++
#include <iostream>
using namespace std;
class Complex {
public:
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
Complex operator+(const Complex& c) const {
return Complex(real + c.real, imag + c.imag);
}
void print() const {
cout << "(" << real << ", " << imag << ")" << endl;
}
private:
double real, imag;
};
int main() {
Complex c1(1, 2), c2(3, 4);
Complex c3 = c1 + c2;
c3.print(); // 输出 (4, 6)
return 0;
}
```
在上面的例子中,我们定义了一个 Complex 类,它包含了实部和虚部两个成员变量,以及一个构造函数和一个打印函数。我们用成员函数 operator+() 重载了加法运算符,使得我们可以使用 c1 + c2 的方式来进行复数加法运算。
阅读全文
相关推荐
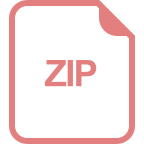
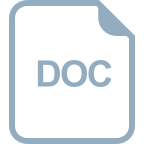
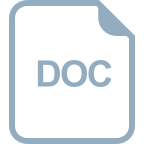
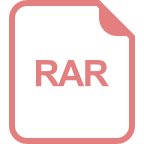
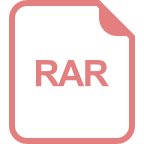
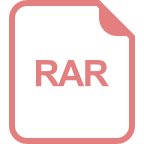
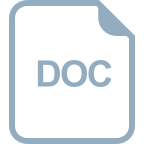
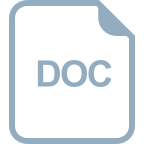
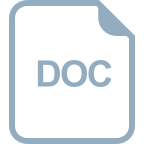
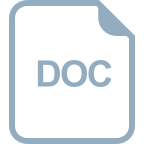
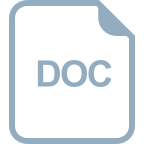
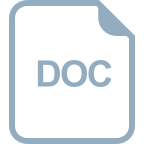
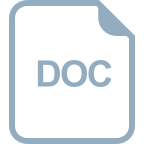
