单链表求最大值c语言
时间: 2024-10-01 13:00:39 浏览: 8
单链表中查找最大值通常需要遍历整个列表,因为不像数组那样可以直接访问元素。以下是一个简单的C语言函数,用于在一个给定的单向链表中找到并返回最大的节点值:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化一个新的链表节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->data = value;
newNode->next = NULL;
}
return newNode;
}
// 找到链表的最大值
int findMax(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
return INT_MIN; // 返回最小整数,表示空链表无最大值
}
int max = head->data;
Node* current = head->next;
while (current != NULL) {
if (current->data > max) {
max = current->data;
}
current = current->next;
}
return max;
}
// 示例用法
int main() {
Node* list = createNode(5);
list->next = createNode(10);
list->next->next = createNode(-3);
list->next->next->next = createNode(20);
int maxVal = findMax(list);
printf("The maximum value in the linked list is: %d\n", maxVal);
return 0;
}
```
在这个示例中,`findMax` 函数首先检查链表是否为空,然后从头节点开始遍历,比较当前节点的值与已知的最大值,并更新最大值。最后返回找到的最大值。
相关推荐
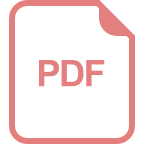
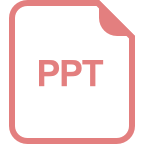
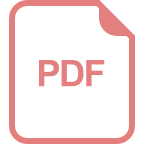














