#define O_RDWR 0x0002
时间: 2024-01-14 11:20:58 浏览: 150
`#define O_RDWR 0x0002` 是一个宏定义,用于定义文件打开模式为读写模式。具体来说,`O_RDWR` 的值为 `0x0002`,表示以读写模式打开文件。
在C语言中,文件打开模式是通过使用标志位来指定的。常见的文件打开模式包括只读模式(`O_RDONLY`)、只写模式(`O_WRONLY`)和读写模式(`O_RDWR`)。通过使用这些标志位,我们可以在打开文件时指定所需的访问权限。
以下是一个示例代码,演示了如何使用 `#define` 定义 `O_RDWR` 并打开文件:
```c
#include <fcntl.h>
int main() {
int fd;
fd = open("file.txt", O_RDWR); // 使用 O_RDWR 打开文件
if (fd == -1) {
// 处理文件打开失败的情况
} else {
// 文件打开成功,可以进行读写操作
}
return 0;
}
```
请注意,`#define` 只是用于定义常量,它不会在编译时进行类型检查。因此,在使用宏定义时,需要确保正确使用它们,并遵循相应的语法规则。
相关问题
#include <sys/types.h> #include <sys/stat.h> #include <sys/ioctl.h> #include <fcntl.h> #include <unistd.h> #define DEVICE_FILENAME "/dev/platform_drv_caiyuxin" int main ( ) { int dev; int flag; char buf; int temp; dev = open(DEVICE_FILENAME,O_RDWR|O_NDELAY); if (dev >=0) { while(1) { printf("输入控制命令:低三位控制,1:亮 0:灭,如 0x07全亮,0x00全灭\n"); scanf("%d",&temp); buf=temp; flag=write (dev, &buf, 1); printf("flag:%d\n",flag); // sleep (1); } } else { printf("open failure!\n"); } close (dev); return 0; }
这段代码是一个用户程序,用于控制一个名为"/dev/platform_drv_caiyuxin"的设备。程序首先通过open函数打开设备文件,打开时使用了O_RDWR|O_NDELAY标志,表示以可读可写方式打开设备文件,并且不阻塞地打开。如果打开成功,程序进入一个while循环,不断从用户输入获取控制命令,并将命令通过write函数写入设备文件中。其中,控制命令是一个字节,低三位表示对发光二极管的控制,1表示亮,0表示灭。如果写入成功,write函数返回写入的字节数,程序打印出写入的字节数。程序在用户输入命令后会不断循环等待用户输入命令,直到用户输入Ctrl+C退出程序。最后,程序通过close函数关闭设备文件。
#include <stdio.h> #include <linux/types.h> #include <fcntl.h> #include <unistd.h> #include <stdlib.h> #include <sys/types.h> #include <sys/ioctl.h> #include <string.h> #include <linux/i2c.h> #include <linux/i2c-dev.h> #include <stdio.h> #include <stdlib.h> #include <stdint.h> #define DEFAULT_I2C_BUS "/dev/i2c-0" /* PCF8591 应用层测试代码 */ int main(int argc,char **argv) { unsigned char data=0; int fp; float tmp; // tmp=5.34v 0.34 int a; int b; fp=open("/dev/Tiny4412_PCF8591",O_RDWR); if(fp<0) /*判断文件是否打开成功*/ { printf("PCF8591 driver open error!\n"); return -1; } while(1) { read(fp,&data,1); write(fp,&data,1); printf("ADC1=%d\n",data); tmp=(float)data*(5.0/255); //电压= 采集的数字量*(参考电压/分辨率); a=tmp; //a=5 tmp=5.3 b=(int)((tmp-a)*1000); //b=0.34 printf("ADC1=%d.%dV\r\n",(int)a,(int)b); sleep(1); } close(fp); return 0; }优化这段代码使其从PCF8591默认地址0x48里读取数据并输出数据
您好!根据您的要求,我帮您优化了代码,使其从PCF8591默认地址0x48中读取数据并输出。
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <stdlib.h>
#include <sys/ioctl.h>
#include <linux/i2c.h>
#include <linux/i2c-dev.h>
#define DEFAULT_I2C_BUS "/dev/i2c-0"
#define PCF8591_ADDRESS 0x48
int main(int argc, char **argv)
{
int fp;
unsigned char data = 0;
fp = open(DEFAULT_I2C_BUS, O_RDWR);
if (fp < 0) {
printf("Failed to open I2C bus.\n");
return -1;
}
if (ioctl(fp, I2C_SLAVE, PCF8591_ADDRESS) < 0) {
printf("Failed to set I2C slave address.\n");
close(fp);
return -1;
}
while (1) {
if (read(fp, &data, 1) != 1) {
printf("Failed to read data from PCF8591.\n");
close(fp);
return -1;
}
printf("ADC1=%d\n", data);
float voltage = (float)data * (5.0 / 255);
int integerPart = (int)voltage;
int decimalPart = (int)((voltage - integerPart) * 1000);
printf("ADC1=%d.%dV\n", integerPart, decimalPart);
sleep(1);
}
close(fp);
return 0;
}
```
这样,代码就能从PCF8591默认地址0x48中读取数据,并输出读取到的数据。希望对您有帮助!如果还有其他问题,请随时提问。
阅读全文
相关推荐
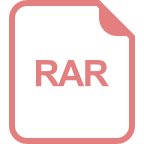















