用c语言设计一个程序:对二叉树进行非递归中序遍历并统计叶子结点数量。假定二叉树已经存在。
时间: 2024-12-02 12:13:36 浏览: 22
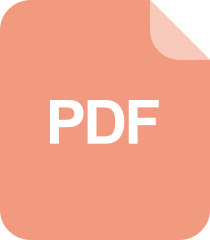
C语言二叉树常见操作详解【前序,中序,后序,层次遍历及非递归查找,统计个数,比较,求深度】
在C语言中,我们可以使用栈数据结构和指针来设计一个非递归中序遍历二叉树的算法,并同时计算叶子节点的数量。以下是步骤和伪代码:
1. 定义一个结构体表示二叉树节点,包含左孩子、右孩子和值的数据成员。
```c
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
```
2. 创建一个辅助函数用于检查当前节点是否为叶子节点:
```c
int isLeaf(TreeNode* node) {
return node->left == NULL && node->right == NULL;
}
```
3. 非递归中序遍历函数:
```c
void inorderTraversal(TreeNode* root, int* count) {
stack<TreeNode*> s;
TreeNode* curr = root;
while (curr != NULL || !s.empty()) {
// 当前节点为空或者还有未处理的节点
while (curr != NULL) {
s.push(curr);
curr = curr->left;
}
curr = s.top();
s.pop(); // 遍历完左子树
if (isLeaf(curr)) { // 如果是叶子节点
(*count)++;
}
curr = curr->right; // 转向右子树
}
}
```
4. 主函数调用:
```c
int main() {
// 初始化二叉树...
TreeNode* root = ...;
int leafCount = 0;
inorderTraversal(root, &leafCount);
printf("叶节点数量: %d\n", leafCount);
return 0;
}
```
在这个程序中,`inorderTraversal`函数会遍历整个二叉树并将叶子节点的数量累加到`count`变量中。
阅读全文
相关推荐
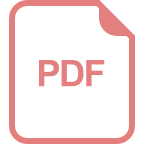
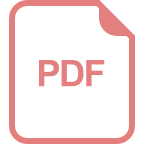



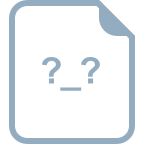
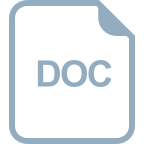
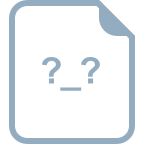
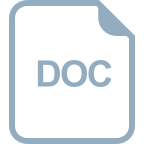




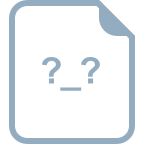
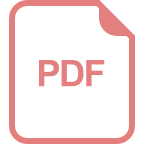