利用c语言求过圆上一点的切线
时间: 2024-09-26 10:00:58 浏览: 59
在C语言中,求解过圆上一点的切线需要一些数学知识,特别是涉及到向量和点到直线的距离公式。假设我们有一个圆心 `center` 和半径 `radius`,以及一个已知点 `point`,想要找出过这个点的切线,我们可以按照以下步骤操作:
1. 首先计算点到圆心的距离 `distance`,如果它等于半径,则说明 `point` 已经在圆上了,那么过该点的切线就是垂直于通过 `point` 的直径。
2. 如果 `distance` 小于半径,我们需要找到两个切点。为此,可以构建一个法向量 `normal`,它是从圆心到点 `point` 的方向向量(圆心减去点),然后取其反向。因为切线的方向应该是垂直于法向量,所以切线向量 `tangent` 可以由 `normal` 乘以一个常数 `t` 来获得,使得 `distance = |tangent|`(注意这将给出两个可能的切线,取决于是否包含原点,可以根据需要选择正确的方向)。
3. 最后,给定一个切线向量,例如 `tangent`,你可以构造切线方程,对于直线 `Ax + By + C = 0`,其中 `(A, B)` 是切线的单位方向向量,`C` 可以通过点 `point` 求得。
以下是简单的伪代码示例:
```c
#include <stdio.h>
#include <math.h>
// 定义结构体表示点
typedef struct {
double x, y;
} Point;
// 点到圆心距离函数
double distance_to_circle(Point center, Point point) {
return sqrt(pow(point.x - center.x, 2) + pow(point.y - center.y, 2));
}
// 计算切线向量
Point tangent_at_point(Point circle_center, Point point) {
Point normal = {circle_center.y - point.y, point.x - circle_center.x};
double distance = distance_to_circle(circle_center, point);
if (fabs(distance - circle.radius) < 1e-6) // 圆上点直接返回垂直直径
return {point.y - circle.center.y, circle.center.x - point.x};
else {
double t = distance / radius; // 根据距离调整t值
return {-normal.y, normal.x}; // 切线方向
}
}
// 主函数示例
int main() {
Point circle_center = {0, 0}, point = {1, 1};
double radius = 1;
Point tangent = tangent_at_point(circle_center, point);
printf("切线斜率: %.2f, y截距: %.2f\n", tangent.x, tangent.y); // 根据需要调整输出格式
return 0;
}
```
阅读全文
相关推荐

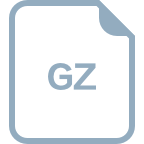