线性表(a1,a2,…,an)中元素递增有序且按顺序存储于计算机内,要求设计一算法完成: (1)用最少时间在表中查找数值为x的元素。 (2)若找到将其与后继元素位置相交换。 (3)若找不到,则将其插入表中并使表中元素仍递增有序。c语言数据结构
时间: 2024-09-29 07:12:12 浏览: 14
这个问题描述的是要在已排序的线性表(数组)中查找、替换或插入一个新值,并保持线性表的递增顺序。这里可以采用二分查找法来提高查找效率。以下是使用 C 语言实现的一个简单算法步骤:
```c
#include <stdio.h>
// 定义结构体表示线性表中的元素
typedef struct {
int value;
struct Node* next;
} Node;
// 插入操作,保持递增有序
void insert(Node** head, int x) {
if (*head == NULL || (*head)->value > x) {
// 如果表为空或者新值小于当前值,插入头部
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->value = x;
newNode->next = *head;
*head = newNode;
} else {
// 否则,在链表中找到合适的位置插入
Node* current = *head;
while (current->next != NULL && current->next->value < x) {
current = current->next;
}
if (current->next != NULL) {
newNode = (Node*)malloc(sizeof(Node));
newNode->value = x;
newNode->next = current->next;
current->next = newNode;
} else {
newNode = (Node*)malloc(sizeof(Node));
newNode->value = x;
newNode->next = NULL;
current->next = newNode; // 插入在最后一个元素之后
}
}
}
// 查找操作,如果找到就交换
void findAndSwap(Node** head, int x, Node** nextToSwap) {
Node* current = *head;
while (current != NULL) {
if (current->value == x) {
// 找到,检查是否需要交换
if (current->next != NULL && current->next->value > x) {
// 跟后继交换
int temp = current->next->value;
current->next->value = x;
x = temp;
*nextToSwap = current->next;
}
break;
}
current = current->next;
}
}
int main() {
Node* head = NULL; // 初始化空表
// 插入和查找操作示例
// ...
return 0;
}
```
相关推荐
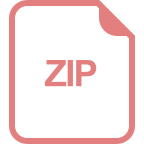
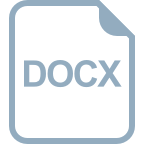
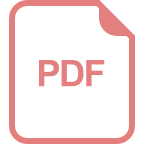



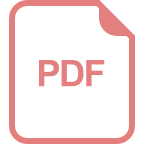
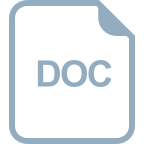
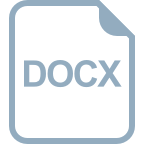
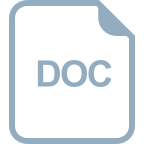
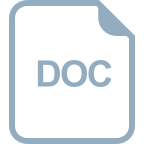
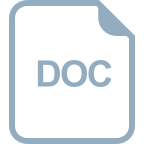
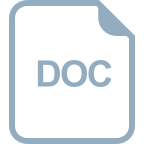
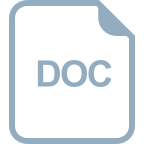
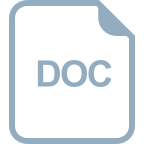
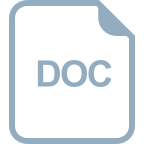
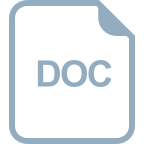