stm32单片机温度控制pid代码
时间: 2023-07-27 10:01:35 浏览: 111
以下是我给出的STM32单片机温度控制PID代码的示例:
#include "stm32f4xx.h"
// 定义温度传感器引脚
#define TEMP_PIN GPIO_Pin_0
#define TEMP_PORT GPIOA
// 定义控制信号引脚
#define CONTROL_PIN GPIO_Pin_1
#define CONTROL_PORT GPIOA
// PID控制器的参数
#define KP 2.0
#define KD 0.5
#define KI 0.1
// 定义一些全局变量
float target_temperature; // 设定温度
float current_temperature; // 当前温度
float previous_error; // 上一次温度误差
float integral_value; // 积分项
// 初始化函数
void PID_Init() {
GPIO_InitTypeDef GPIO_InitStruct;
// 初始化温度传感器引脚
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE); // 使能端口A时钟
GPIO_InitStruct.GPIO_Pin = TEMP_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AN; // 模拟输入
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL; // 不使用上下拉电阻
GPIO_Init(TEMP_PORT, &GPIO_InitStruct);
// 初始化控制信号引脚
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE); // 使能端口A时钟
GPIO_InitStruct.GPIO_Pin = CONTROL_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_OUT; // 输出
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP; // 推挽输出
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_100MHz; // 最大速度100MHz
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL; // 不使用上下拉电阻
GPIO_Init(CONTROL_PORT, &GPIO_InitStruct);
// 初始化一些全局变量
target_temperature = 0;
current_temperature = 0;
previous_error = 0;
integral_value = 0;
}
// 读取当前温度值的函数
float ReadTemperature() {
// 读取温度传感器的模拟信号并转换为温度值
// 这里需要根据实际情况编写相应的代码
// 然后返回当前温度值
}
// 控制输出函数
void ControlOutput(float output) {
// 将控制信号写入控制信号引脚
// 这里需要根据实际情况编写相应的代码
// 比如使用PWM控制信号来控制加热元件的功率
}
// PID控制函数
void PID_Control() {
// 读取当前温度值
current_temperature = ReadTemperature();
// 计算温度误差
float error = target_temperature - current_temperature;
// 计算PID控制输出
float proportional = KP * error;
float derivative = KD * (error - previous_error);
float integral = KI * integration_value;
float output = proportional + derivative + integral;
// 更新一些全局变量
previous_error = error;
integration_value += error;
// 控制输出
ControlOutput(output);
}
int main(void) {
// 初始化PID控制器
PID_Init();
while (1) {
// 进行PID控制
PID_Control();
}
}
希望这个示例对您有所帮助!请注意,该代码仅供参考,您可能需要根据您的具体硬件和需求进行适当的修改和调整。
相关推荐
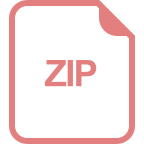














