msgsnd:向消息队列发送消息。
时间: 2024-06-14 12:09:13 浏览: 159
msgsnd是一个系统调用函数,用于向消息队列发送消息。它的原型如下:
```c
int msgsnd(int msqid, const void *msgp, size_t msgsz, int msgflg);
```
参数说明:
- msqid:消息队列的标识符,通过msgget函数获取。
- msgp:指向要发送的消息的指针。
- msgsz:要发送的消息的大小(以字节为单位)。
- msgflg:控制发送消息的行为,可以使用IPC_NOWAIT标志来指定非阻塞模式。
msgsnd函数将消息添加到消息队列的末尾,并根据消息类型进行排序。如果消息队列已满,且没有指定IPC_NOWAIT标志,则msgsnd函数将阻塞,直到有空间可用为止。如果指定了IPC_NOWAIT标志,则msgsnd函数将立即返回,并返回-1作为错误码。
注意事项:
- 消息队列中的消息是按照先进先出(FIFO)的顺序进行处理。
- 发送的消息可以是任意类型的结构体,但是需要保证接收方能够正确解析和处理。
相关问题
1) 编写C程序,利用msgget以及msgsnd完成消息队列的创建及向消息队列发送消息。
以下是一个简单的示例程序,可以创建一个消息队列,并向其中发送一条消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MSG_SIZE 1024
struct message {
long mtype;
char mtext[MSG_SIZE];
};
int main(int argc, char *argv[]) {
key_t key;
int msgid;
struct message msg;
// 创建一个唯一的key,用于标识消息队列
key = ftok(".", 'a');
if (key == -1) {
perror("ftok");
exit(EXIT_FAILURE);
}
// 创建一个消息队列,如果已经存在则直接打开
msgid = msgget(key, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 填充消息内容
msg.mtype = 1;
strncpy(msg.mtext, "Hello, message queue!", MSG_SIZE);
// 发送消息到队列中
if (msgsnd(msgid, &msg, sizeof(msg.mtext), 0) == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
printf("Message sent: %s\n", msg.mtext);
return 0;
}
```
在上述程序中,首先使用 `ftok` 函数生成一个唯一的 key 值,然后使用 `msgget` 函数创建或打开一个消息队列。接下来,定义了一个 `struct message` 结构体,用于存储要发送的消息。填充消息内容后,使用 `msgsnd` 函数将消息发送到队列中。最后,打印出发送的消息内容。
注意,当使用 `msgget` 函数创建消息队列时,需要指定权限参数,例如 `0666`,表示创建的消息队列对所有用户可读可写。如果使用已存在的消息队列,则可以省略该参数。
运行程序后,可以使用 `ipcs -q` 命令查看系统中存在的消息队列。可以看到刚刚创建的消息队列:
```
$ ipcs -q
------ Message Queues --------
key msqid owner perms used-bytes messages
0x61020161 98304 user 666 31 1
```
可以使用 `ipcrm -q` 命令删除指定的消息队列。例如,要删除上述示例程序创建的消息队列,可以执行以下命令:
```
$ ipcrm -q 98304
```
完成以下程序:进程A向消息队列发送消息“hello,world” 进程B从消息队列读取消息,并打印。 进程C向消息队列发送“自己在姓名” 进程D从消息队列中取出姓名字符串,并打印
下面是基于Linux系统下的C语言代码实现:
进程A发送消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/msg.h>
#define MSG_SIZE 256
typedef struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
} msg_buf;
int main() {
int msgid;
msg_buf msg;
// 创建消息队列
msgid = msgget(IPC_PRIVATE, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget error");
exit(EXIT_FAILURE);
}
// 发送消息
msg.mtype = 1;
strcpy(msg.mtext, "hello, world");
if (msgsnd(msgid, &msg, sizeof(msg.mtext), 0) == -1) {
perror("msgsnd error");
exit(EXIT_FAILURE);
}
return 0;
}
```
进程B接收消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/msg.h>
#define MSG_SIZE 256
typedef struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
} msg_buf;
int main() {
int msgid;
msg_buf msg;
// 获取消息队列
msgid = msgget(IPC_PRIVATE, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget error");
exit(EXIT_FAILURE);
}
// 接收消息
if (msgrcv(msgid, &msg, sizeof(msg.mtext), 1, 0) == -1) {
perror("msgrcv error");
exit(EXIT_FAILURE);
}
printf("%s\n", msg.mtext);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl error");
exit(EXIT_FAILURE);
}
return 0;
}
```
进程C发送姓名消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/msg.h>
#define MSG_SIZE 256
typedef struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
} msg_buf;
int main() {
int msgid;
msg_buf msg;
// 创建消息队列
msgid = msgget(IPC_PRIVATE, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget error");
exit(EXIT_FAILURE);
}
// 发送消息
msg.mtype = 1;
strcpy(msg.mtext, "Jack");
if (msgsnd(msgid, &msg, sizeof(msg.mtext), 0) == -1) {
perror("msgsnd error");
exit(EXIT_FAILURE);
}
return 0;
}
```
进程D接收姓名消息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/msg.h>
#define MSG_SIZE 256
typedef struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
} msg_buf;
int main() {
int msgid;
msg_buf msg;
// 获取消息队列
msgid = msgget(IPC_PRIVATE, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget error");
exit(EXIT_FAILURE);
}
// 接收消息
if (msgrcv(msgid, &msg, sizeof(msg.mtext), 1, 0) == -1) {
perror("msgrcv error");
exit(EXIT_FAILURE);
}
printf("%s\n", msg.mtext);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl error");
exit(EXIT_FAILURE);
}
return 0;
}
```
以上代码实现了进程间通过消息队列进行通信的功能,具体的原理和实现细节请参考Linux系统下的相关文档。
阅读全文
相关推荐
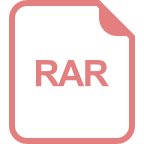
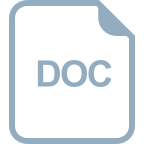
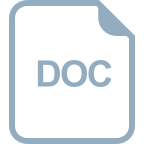
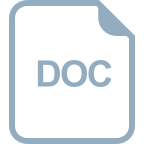
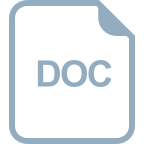
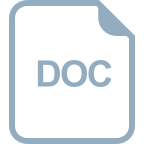
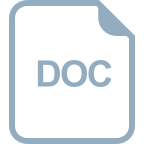





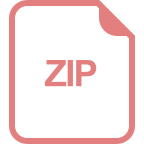
