springboot调用第三方api接口
时间: 2024-09-10 11:28:40 浏览: 88
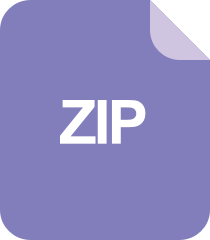
SpringBoot使用Feign调用第三方接口Demo.zip
Spring Boot调用第三方API接口通常会用到一些常用的Java库,比如Apache HttpClient、OkHttp或者Spring提供的RestTemplate。下面是使用RestTemplate进行API调用的基本步骤:
1. 引入依赖:在Spring Boot项目的pom.xml中添加`spring-boot-starter-web`依赖,这个依赖中包含了RestTemplate。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
2. 注入RestTemplate:在Spring Boot的主类或配置类上添加`@SpringBootApplication`注解,并在需要使用RestTemplate的类中使用`@Autowired`注解来注入RestTemplate实例。
```java
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
public class SomeService {
@Autowired
private RestTemplate restTemplate;
// 其他代码...
}
```
3. 调用第三方API:在业务逻辑中使用注入的RestTemplate调用第三方API接口。可以通过GET、POST、PUT、DELETE等方法发送HTTP请求,并处理返回的结果。
```java
public String callThirdPartyApi(String apiUrl, Map<String, String> params) {
// 如果API需要参数,可以使用MultiValueMap构建请求参数
MultiValueMap<String, String> map = new LinkedMultiValueMap<>();
map.setAll(params);
// 发送GET请求
ResponseEntity<String> response = restTemplate.getForEntity(apiUrl, String.class, map);
// 处理响应
return response.getBody();
}
```
4. 错误处理和配置:在调用第三方API时,需要处理可能出现的异常,如`HttpClientErrorException`或`HttpServerErrorException`。还可以配置RestTemplate,例如自定义连接超时、读取超时等。
阅读全文
相关推荐
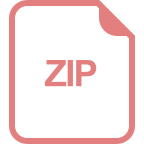
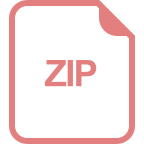











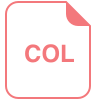



