java springboot项目如何调用第三方接口
时间: 2024-09-13 13:10:38 浏览: 67
在Java Spring Boot项目中调用第三方接口通常涉及以下几个步骤:
1. **添加依赖**:首先,你需要在项目的pom.xml文件中添加对第三方API库的依赖。例如,如果你要调用的是HTTP RESTful API,可以使用`spring-boot-starter-web`或者`com.squareup.retrofit2:retrofit`等库。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- 或者使用Retrofit -->
<dependency>
<groupId>com.squareup.retrofit2</groupId>
<artifactId>retrofit</artifactId>
</dependency>
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
</dependency>
```
2. **配置RestTemplate或Retrofit**:Spring Boot提供了`RestTemplate`用于发送HTTP请求,而Retrofit则是一个强大的客户端库。以下是配置Retrofit的例子:
```java
@Configuration
public class ApiServiceConfig {
@Bean
public OkHttpClient httpClient() {
return new OkHttpClient();
}
@Bean
public Retrofit retrofit(OkHttpClient client) {
return new Retrofit.Builder()
.baseUrl("https://api.example.com/")
.addConverterFactory(GsonConverterFactory.create())
.client(client)
.build();
}
@Bean
public YourApiService yourApiService(Retrofit retrofit) {
return retrofit.create(YourApiService.class);
}
}
```
其中,`YourApiService`是你自定义的接口类,对应第三方API的公共方法。
3. **调用接口**:有了服务实例后,你可以通过注入到需要使用的类中,然后像操作本地方法一样调用它:
```java
@Service
public class YourService {
private final YourApiService apiService;
@Autowired
public YourService(YourApiService apiService) {
this.apiService = apiService;
}
public void fetchData() {
Call<ApiResponse> call = apiService.getSomeData();
call.enqueue(new Callback<ApiResponse>() {
// 处理响应...
});
}
}
```
阅读全文
相关推荐
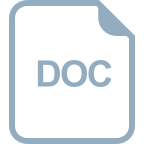
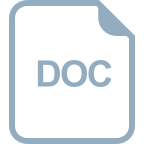
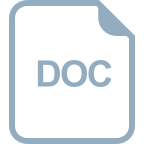
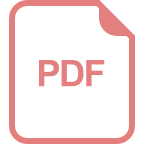








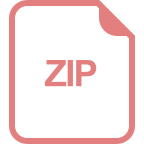





