springboot通过http调用第三方接口
时间: 2024-09-06 20:04:27 浏览: 101
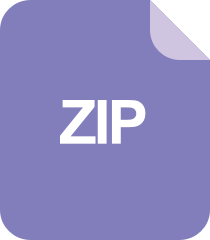
SpringBoot使用Feign调用第三方接口Demo.zip
Spring Boot通过HTTP调用第三方接口通常涉及以下几个步骤:
1. 添加依赖:在Spring Boot项目的`pom.xml`或`build.gradle`文件中添加HTTP客户端库的依赖,比如常用的`RestTemplate`或`WebClient`。
2. 创建HTTP客户端:可以使用Spring提供的`RestTemplate`或`WebClient`来创建HTTP请求。
3. 配置HTTP客户端:根据需要配置HTTP客户端的相关参数,比如连接超时时间、读取超时时间等。
4. 发起HTTP请求:通过配置好的HTTP客户端对象发起GET、POST等HTTP请求。
5. 处理响应:对第三方接口返回的响应进行解析和处理。
下面是一个使用`RestTemplate`发起GET请求的简单示例:
```java
import org.springframework.web.client.RestTemplate;
public class ThirdPartyApiCaller {
private RestTemplate restTemplate = new RestTemplate();
public String callThirdPartyApi(String url) {
String response = restTemplate.getForObject(url, String.class);
return response;
}
}
```
使用`WebClient`发起GET请求的示例:
```java
import org.springframework.web.reactive.function.client.WebClient;
public class ThirdPartyApiCaller {
private WebClient webClient = WebClient.create();
public Mono<String> callThirdPartyApi(String url) {
return webClient.get()
.uri(url)
.retrieve()
.bodyToMono(String.class);
}
}
```
在Spring Boot应用中,通常需要在服务启动类上添加`@EnableWebMvc`注解来启用Spring MVC支持,或者在`application.properties`/`application.yml`配置文件中添加相关配置。
阅读全文
相关推荐
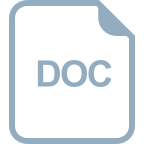
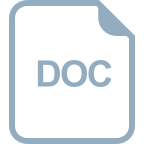

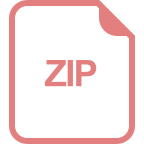
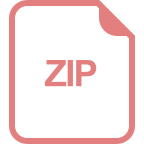








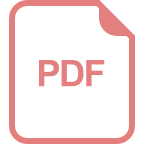



