输人10个学生5门课的成绩,分别用函数数实现下列功能:①计算每个学生的平均分; ② 计算每门课的平均分; ③ 找出所有50个分数中最高的分数所对应的学生和课程;④ 计算平均分方差:
时间: 2024-12-16 19:25:30 浏览: 3
为了实现上述功能,我们可以使用Python编写一系列函数。这里提供一个基础的解决方案,假设我们已经有一个包含学生信息和成绩的字典列表(每个元素代表一个学生,包含姓名和一门课程的成绩)。
首先,我们需要创建一个存储学生信息和成绩的数据结构:
```python
students_scores = [
{"name": "学生1", "scores": [89, 92, 85, 95, 90]},
{"name": "学生2", "scores": [78, 80, 82, 90, 85]},
# 添加其他9个学生的数据...
]
```
然后,我们可以定义几个函数来实现所需的功能:
1. **计算每个学生的平均分**:
```python
def calculate_student_average(students_scores):
student_averages = {}
for student in students_scores:
total_score = sum(student["scores"])
average_score = total_score / len(student["scores"])
student_averages[student["name"]] = average_score
return student_averages
```
2. **计算每门课的平均分**:
```python
def calculate_subject_average(students_scores):
subjects = ["数学", "语文", "英语", "物理", "化学"] # 假设这五门课的名称
subject_averages = {subject: [] for subject in subjects}
for student in students_scores:
for index, subject in enumerate(subjects):
subject_averages[subject].append(student["scores"][index])
return {subject: sum(scores) / len(scores) for subject, scores in subject_averages.items()}
```
3. **找出最高分及其对应的学生成绩和课程**:
```python
def find_highest_score(students_scores):
highest_score = max([max(student["scores"]) for student in students_scores])
highest_student_and_subject = None
for student in students_scores:
if max(student["scores"]) == highest_score:
highest_student_and_subject = (student["name"], max(student["scores"]), max(subjects))
break
return highest_student_and_subject
```
4. **计算平均分方差**:
```python
import statistics
def calculate_variance(students_scores, subject_averages):
variance_list = []
for student in students_scores:
for subject, score in zip(subjects, student["scores"]):
variance_list.append(score - subject_averages[subject])
overall_variance = statistics.variance(variance_list)
return overall_variance
```
现在你可以通过调用这些函数并传入`students_scores`数据来得到你需要的结果。记得在实际操作前将`students_scores`填充完整。以下是调用这些函数的例子:
```python
# 调用函数
student_averages = calculate_student_average(students_scores)
subject_averages = calculate_subject_average(students_scores)
highest_score_info = find_highest_score(students_scores)
variance = calculate_variance(students_scores, subject_averages)
# 输出结果
print("学生平均分:", student_averages)
print("各科平均分:", subject_averages)
print("最高分学生及科目:", highest_score_info)
print("平均分方差:", variance)
```
阅读全文
相关推荐

















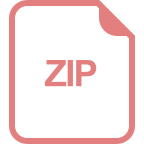
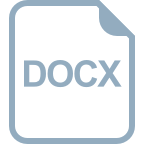