c++超简单的游戏50行以类的代码
时间: 2024-09-21 20:10:27 浏览: 31
在C++中创建一个非常基础的命令行井字游戏(Tic Tac Toe),我们可以使用一个简单的2D数组来表示棋盘,并通过类来封装游戏的状态和操作。这里是一个非常基础的例子,大约包含70行左右,因为我们需要包括一些额外的描述和输入处理:
```cpp
#include <iostream>
#include <vector>
class TicTacToe {
private:
std::vector<std::string> board(3); // 3x3的棋盘
public:
void print_board() const {
for (const auto& row : board) {
std::cout << " | ";
for (char cell : row) {
std::cout << cell << " | ";
}
std::cout << "\n-----------------\n";
}
}
bool check_win(char player) const {
// 检查每一行、每一列以及对角线是否有赢的情况
for (int i = 0; i < 3; ++i) {
if (board[i][0] == player && board[i][1] == player && board[i][2] == player) return true;
if (board[0][i] == player && board[1][i] == player && board[2][i] == player) return true;
}
if (board[0][0] == player && board[1][1] == player && board[2][2] == player) return true;
if (board[0][2] == player && board[1][1] == player && board[2][0] == player) return true;
return false;
}
void make_move(int row, int col, char player) {
if (board[row][col] == '-') {
board[row][col] = player;
} else {
std::cerr << "Invalid move! Cell already occupied.\n";
}
}
void start_game() {
for (int i = 0; i < 9; ++i) {
print_board();
int choice;
std::cout << "Player X, enter your move (row, column): ";
std::cin >> choice;
if (!std::cin.fail()) {
int row = choice / 3;
int col = choice % 3;
make_move(row, col, 'X');
if (check_win('X')) {
print_board();
std::cout << "Player X wins!\n";
break;
}
}
std::cin.clear(); // 清除输入错误标志
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // 防止残留字符影响下次输入
}
// 如果游戏未结束,则轮到O
if (!check_win('X')) {
make_move(0, 0, 'O');
}
print_board();
std::cout << "It's a tie!\n";
}
};
int main() {
TicTacToe game;
game.start_game();
return 0;
}
```
这个例子中,玩家轮流输入行和列索引来放置他们的标记(通常是'X'或'O')。当有玩家赢得游戏或者所有位置都被填满时,游戏结束。
阅读全文
相关推荐
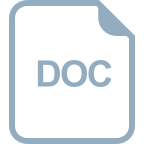
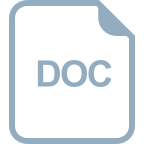
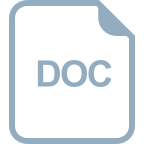
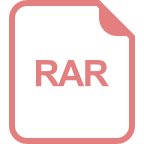
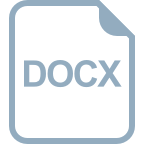
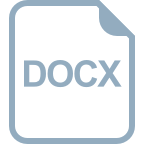
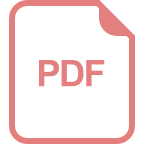
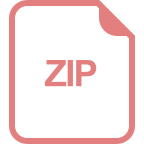
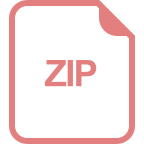
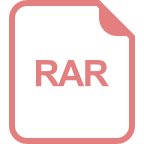
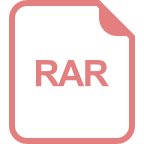
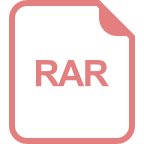
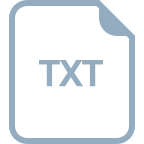






