按照上述要求,建立一级菜单A,B,C。建立他们的二级菜单分别为A1,A2,A3 B1,B2,B3 C1,C2,C3。完善代码,写一个成品代码
时间: 2024-10-10 17:06:48 浏览: 38
为了满足您的需求,我会给出一个完整的C语言代码示例,它包含了主菜单、子菜单以及用户输入处理。请注意,这个例子假设我们使用字符变量来代表菜单选项,而不是真正的子菜单对象,因为实际项目中可能需要更复杂的数据结构。
```c
#include <stdio.h>
#include <stdlib.h>
enum MenuLevel { LEVEL_1, LEVEL_2 };
enum SubMenu { SUBMENU_A1, SUBMENU_A2, SUBMENU_A3,
SUBMENU_B1, SUBMENU_B2, SUBMENU_B3,
SUBMENU_C1, SUBMENU_C2, SUBMENU_C3 };
// 存储菜单信息的结构体
struct MenuEntry {
char level;
char submenu;
struct MenuEntry* next;
};
// 创建一级菜单并添加子菜单
void createMenu(struct MenuEntry** root, int level, int submenu) {
struct MenuEntry* new_entry = (struct MenuEntry*)malloc(sizeof(struct MenuEntry));
new_entry->level = level;
new_entry->submenu = (char)('A' + submenu - 1); // 对应字母编号
new_entry->next = NULL;
if (*root == NULL) {
*root = new_entry;
} else {
struct MenuEntry* prev = *root;
while (prev->next != NULL && prev->next->level == level) {
prev = prev->next;
}
prev->next = new_entry;
}
}
// 显示菜单
void displayMenu(struct MenuEntry* root) {
while (root != NULL) {
printf("Level %d: %c\n", root->level, root->submenu);
root = root->next;
}
}
int main() {
struct MenuEntry* root = NULL;
// 创建一级菜单
createMenu(&root, LEVEL_1, 1); // A
createMenu(&root, LEVEL_1, 2); // B
createMenu(&root, LEVEL_1, 3); // C
// 添加每个一级菜单的二级菜单
createMenu(root->next, LEVEL_2, 1); // A1
// ... (依次为其他子菜单)
// 显示菜单
displayMenu(root);
// 用户输入处理示例,这里简化为模拟用户选择
char choice;
do {
printf("\nEnter your choice (A[1-3], B[1-3], C[1-3]): ");
scanf("%c", &choice);
// 简化处理,假设用户输入的是数字对应的一级菜单
int menu_index = choice - 'A';
if (menu_index >= 1 && menu_index <= 3) {
struct MenuEntry* current = root + menu_index - 1;
if (current->level == 2) {
printf("You are in Level 2. Choose a sub-menu: ");
// 更详细处理,如读取子菜单编号
} else {
displayMenu(current);
}
} else {
printf("Invalid input. Please try again.\n");
}
} while (choice != '\n'); // 结束条件可以自定义
free(root);
return 0;
}
```
注意:这只是一个基本的框架,实际应用中还需要对用户输入进行更精确的解析,并添加错误处理和用户交互逻辑。以上代码仅供参考,根据具体需求进行调整。
阅读全文
相关推荐
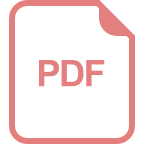
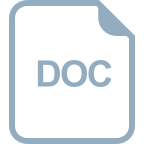
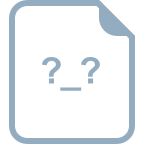
















