有城市S、A1、A2、A3、B1、B2、C1、C2、T,他们之间的距离如下: S-A1:6 T-A2:3 S-A3:3 A1-B1:6 A1-B2:5 A2-B1:6 A2-B2:6 A3-B1:7 A3-B2:4 B1-C1:7 B1-C2:7 B2-C1:8 B2-C2:9 C1-T:5 C2-T:6 现在要求让一条路径同时经过S、A1、A2、A3、B1、B2、C1、C2、T,请用Python代码求解最短路径及路径的长度。
时间: 2023-07-10 20:32:24 浏览: 56
这是一个最短路径问题,可以使用Dijkstra算法或者Floyd算法进行求解。以下是使用Dijkstra算法的示例代码:
```python
import heapq
# 构建有向图
graph = {
'S': {'A1': 6, 'A3': 3},
'A1': {'S': 6, 'A2': 3, 'A3': 6, 'B1': 5, 'B2': 4},
'A2': {'A1': 3, 'B1': 6, 'B2': 6, 'C1': 7, 'C2': 8, 'T': 3},
'A3': {'S': 3, 'A1': 6, 'B1': 7, 'B2': 4},
'B1': {'A1': 5, 'A2': 6, 'A3': 7, 'B2': 7, 'C1': 7, 'C2': 8},
'B2': {'A1': 4, 'A2': 6, 'A3': 4, 'B1': 7, 'C1': 8, 'C2': 9},
'C1': {'B1': 7, 'B2': 8, 'A2': 7, 'C2': 5, 'T': 5},
'C2': {'B1': 8, 'B2': 9, 'A2': 8, 'C1': 5, 'T': 6},
'T': {'A2': 3, 'C1': 5, 'C2': 6}
}
def dijkstra(graph, start, end):
# 初始化距离列表和堆
distances = {node: float('inf') for node in graph}
distances[start] = 0
heap = [(0, start)]
# 初始化前驱节点列表
previous_nodes = {node: None for node in graph}
while heap:
# 弹出堆中距离最小的节点
(distance, current_node) = heapq.heappop(heap)
# 如果该节点已经访问过,则跳过
if distance > distances[current_node]:
continue
# 遍历该节点的所有邻居
for neighbor, weight in graph[current_node].items():
new_distance = distance + weight
# 如果新的距离比之前的距离更小,则更新距离和前驱节点
if new_distance < distances[neighbor]:
distances[neighbor] = new_distance
previous_nodes[neighbor] = current_node
heapq.heappush(heap, (new_distance, neighbor))
# 构建路径
path = []
current_node = end
while current_node is not None:
path.insert(0, current_node)
current_node = previous_nodes[current_node]
return distances[end], path
start = 'S'
end = 'T'
distance, path = dijkstra(graph, start, end)
print(f'最短路径为:{" -> ".join(path)},长度为:{distance}')
```
在上述代码中,我们首先构建了一个有向图,然后使用Dijkstra算法求解经过指定节点的最短路径。其中,使用了堆来维护当前距离最小的节点,以提高算法的效率。最后,输出了最短路径及其长度。
相关推荐
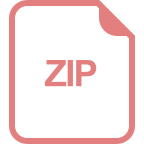
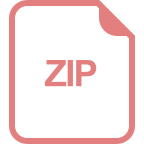
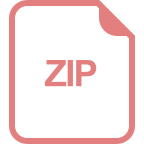














