Python人脸-性别识别代码
时间: 2024-06-24 21:01:10 浏览: 9
在Python中,使用人脸识别技术并结合性别识别通常涉及到深度学习库,如OpenCV、Dlib、Face_recognition和TensorFlow等。以下是一个简单的示例,展示如何使用dlib库进行人脸检测,并使用性别预测模型(例如基于机器学习的模型)来预测性别:
```python
# 导入必要的库
import cv2
import dlib
from PIL import Image
import numpy as np
# 下载并加载预训练的人脸检测模型(Haar级联分类器)
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
# 下载并加载预训练的性别预测模型(假设是一个sklearn模型,例如使用Iris数据集中的特征和性别作为标签)
# 这里只是一个假设,实际可能需要使用更复杂的模型,如face_recognition中的卷积神经网络模型
gender_model = load_gender_model() # 假设函数load_gender_model()已经定义好了模型加载
def detect_face_and_predict_gender(image_path):
# 加载图像
img = cv2.imread(image_path)
# 转换为灰度图像以便于人脸检测
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = face_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5)
for (x, y, w, h) in faces:
# 提取人脸区域
face_roi = img[y:y+h, x:x+w]
# 将图像转换为PIL格式,因为dlib需要这种格式
pil_image = Image.fromarray(face_roi)
# 将PIL图像调整为模型所需的尺寸
resized_image = pil_image.resize((48, 48)) # 假设你的模型需要48x48像素的输入
# 将numpy数组转换回图像
face_array = np.array(resized_image)
# 预测性别
gender_pred = gender_model.predict([face_array])
# 在原始图像上标注性别预测结果
cv2.putText(img, f"Gender: {gender_pred}", (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
# 显示结果
cv2.imshow("Gender Detection", img)
cv2.waitKey(0)
# 使用方法
detect_face_and_predict_gender('path_to_your_image.jpg')
```
请注意,这个示例是简化版本,实际应用中你可能需要更复杂的数据预处理和模型优化。此外,对于精确的性别识别,面部关键点的位置和角度对结果有很大影响,因此人脸对齐预处理也是必要的。
相关推荐
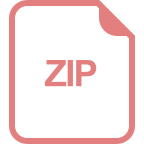
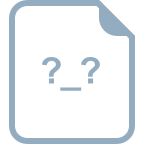
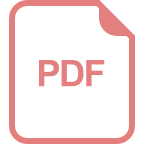














