nsga-ii多目标优化的代码
时间: 2024-05-02 18:15:03 浏览: 14
NSGA-II(Non-dominated Sorting Genetic Algorithm II)是一种常用的多目标优化算法。它基于遗传算法的思想,通过遗传操作和非支配排序来搜索多目标优化问题的解集。
以下是NSGA-II多目标优化算法的代码实现示例:
```python
# 导入所需的库
import random
# 定义个体类
class Individual:
def __init__(self, chromosome):
self.chromosome = chromosome
self.fitness = None
self.rank = None
self.crowding_distance = None
# 定义NSGA-II算法类
class NSGA2:
def __init__(self, population_size, num_generations, crossover_rate, mutation_rate):
self.population_size = population_size
self.num_generations = num_generations
self.crossover_rate = crossover_rate
self.mutation_rate = mutation_rate
def initialize_population(self):
population = []
for _ in range(self.population_size):
chromosome = [random.randint(0, 1) for _ in range(num_variables)]
individual = Individual(chromosome)
population.append(individual)
return population
def evaluate_population(self, population):
for individual in population:
# 计算个体的适应度值
individual.fitness = evaluate_fitness(individual.chromosome)
def non_dominated_sort(self, population):
# 实现非支配排序算法
# ...
def crowding_distance_assignment(self, population):
# 实现拥挤度距离计算算法
# ...
def selection(self, population):
# 实现选择操作,如锦标赛选择
# ...
def crossover(self, parent1, parent2):
# 实现交叉操作,如单点交叉
# ...
def mutation(self, individual):
# 实现变异操作,如位翻转变异
# ...
def evolve(self):
population = self.initialize_population()
self.evaluate_population(population)
for generation in range(self.num_generations):
offspring = []
while len(offspring) < self.population_size:
parent1 = self.selection(population)
parent2 = self.selection(population)
if random.random() < self.crossover_rate:
child1, child2 = self.crossover(parent1, parent2)
offspring.append(child1)
offspring.append(child2)
else:
offspring.append(parent1)
offspring.append(parent2)
for individual in offspring:
if random.random() < self.mutation_rate:
self.mutation(individual)
self.evaluate_population(offspring)
population += offspring
fronts = self.non_dominated_sort(population)
population = []
for front in fronts:
self.crowding_distance_assignment(front)
population += front
if len(population) >= self.population_size:
break
return population
# 示例使用
num_variables = 10
population_size = 100
num_generations = 50
crossover_rate = 0.8
mutation_rate = 0.1
nsga2 = NSGA2(population_size, num_generations, crossover_rate, mutation_rate)
final_population = nsga2.evolve()
# 输出最终种群中的个体及其适应度值
for individual in final_population:
print(individual.chromosome, individual.fitness)
```
以上是一个简单的NSGA-II多目标优化算法的代码实现示例,其中涉及到个体类的定义、种群初始化、适应度评估、非支配排序、拥挤度距离计算、选择、交叉、变异等操作。具体的适应度评估、非支配排序和拥挤度距离计算等函数需要根据具体问题进行实现。
相关推荐
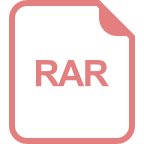












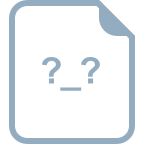
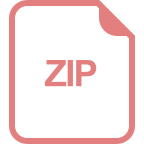