python读取csv文件并去除特殊符号¥
时间: 2024-08-14 18:08:42 浏览: 126
在Python中,你可以使用pandas库方便地读取CSV文件,并对数据进行预处理,比如移除特殊字符。以下是一个简单的步骤:
首先,确保你已经安装了pandas和openpyxl(如果文件是Excel格式,需要这个)。如果没有,可以使用pip install pandas openpyxl命令安装。
```python
import pandas as pd
# 读取CSV文件
df = pd.read_csv('your_file.csv')
# 定义要去除的特殊字符列表,这里以"¥"为例
special_chars = ['¥']
# 使用str.replace()函数替换特定字符
for char in special_chars:
df = df.applymap(lambda x: x.strip(char) if isinstance(x, str) else x)
# 保存处理后的数据到新的CSV文件
df.to_csv('new_file.csv', index=False)
```
这段代码会遍历DataFrame中的每一列(如果是字符串),将其中的"¥"字符替换为空。如果你只是想保留某些列,可以在applymap之前添加一个条件判断。
相关问题
python语言利用pandas删除csv文件第一列并且删除重复行,再对其中"评论内容"进行预料清洗
可以使用以下代码实现:
```python
import pandas as pd
import re
# 读取csv文件并删除第一列
df = pd.read_csv('filename.csv', usecols=range(1, len(df.columns)))
# 删除重复行
df.drop_duplicates(inplace=True)
# 定义文本预处理函数
def clean_text(text):
# 去除标点符号、数字和特殊字符
text = re.sub(r'[^\w\s]', '', text)
text = re.sub(r'\d+', '', text)
text = re.sub(r'\s+', ' ', text)
text = text.strip()
return text.lower()
# 对评论内容进行预处理
df['评论内容'] = df['评论内容'].apply(clean_text)
# 保存处理后的数据到新的csv文件
df.to_csv('new_filename.csv', index=False)
```
其中,`filename.csv` 是要处理的原始csv文件的文件名,`new_filename.csv` 是处理后的新文件的文件名。`clean_text()` 函数用于对文本进行预处理,去除标点符号、数字和特殊字符,并将文本转换为小写。最后,将处理后的数据保存到新的csv文件中。
python数据预处理。从文件中读取数据到DataFrame中,对手机评论数据利用jieba模块进行分词、去除停用词等进行处理。
好的,我可以为您解答这个问题。
首先,我们需要导入需要的库和模块:
```python
import pandas as pd
import jieba
from jieba import analyse
import re
```
接下来,我们可以读取数据文件(假设为csv格式),将其转换成DataFrame类型:
```python
data = pd.read_csv('data.csv')
```
然后,我们可以对数据进行处理。首先,我们需要定义一个函数,用于分词、去除停用词等处理:
```python
def clean_text(text):
# 使用正则表达式去除标点符号和特殊字符
text = re.sub('[^\w\s]', '', text)
# 分词
seg_list = jieba.cut(text, cut_all=False)
# 去除停用词
stopwords = [line.strip() for line in open('stopwords.txt', 'r', encoding='utf-8').readlines()] # 读取停用词表
seg_list = [word for word in seg_list if word not in stopwords]
# 返回处理后的结果
return ' '.join(seg_list)
```
其中,stopwords.txt是一个存储停用词的文件,需要事先准备好。
接下来,我们可以使用apply方法将该函数应用到所有评论数据上:
```python
data['cleaned_text'] = data['text'].apply(clean_text)
```
最后,我们可以将处理后的数据保存到文件中:
```python
data.to_csv('cleaned_data.csv', index=False)
```
这样,我们就完成了对手机评论数据的预处理,得到了一个干净的、可以用于后续分析的数据集。
阅读全文
相关推荐
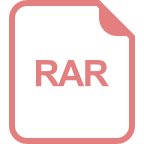
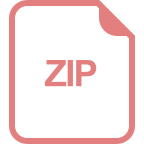
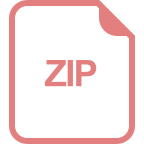
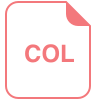
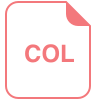
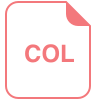
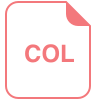
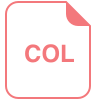
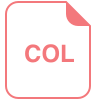
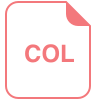
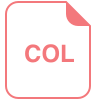
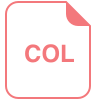
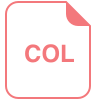
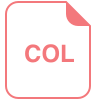


