上位机串口通讯怎么写
时间: 2024-08-19 16:00:36 浏览: 58
上位机串口通信是一种常见的数据传输方式,通常用于微控制器、嵌入式设备与PC机之间的通信。在编写上位机串口通信程序时,可以遵循以下步骤:
1. 初始化串口:根据通信参数(如波特率、数据位、停止位和校验位)设置串口,确保通信双方的设置一致。
2. 打开串口:通过系统调用函数打开串口设备文件,例如在Windows中使用CreateFile(),在Linux中使用open()。
3. 配置串口属性:根据需要配置串口的各种属性,如读写超时、缓冲区大小等。
4. 读写串口:使用read()和write()函数对串口进行数据的读取和发送。在Windows中可能需要使用ReadFile()和WriteFile()。
5. 关闭串口:通信结束后,应该关闭串口以释放资源,使用CloseHandle()在Windows中,或者使用close()在Linux中。
6. 异常处理:对于可能出现的错误,如读写超时、设备不存在等,应该进行相应的错误处理。
7. 同步或异步操作:根据实际需求选择同步读写或使用事件驱动的异步读写。
这里是一个在Linux环境下使用C语言编写上位机串口通信的简单示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main() {
int serial_port = open("/dev/ttyS0", O_RDWR);
if (serial_port < 0) {
printf("Error %i from open: %s\n", errno, strerror(errno));
return 1;
}
// 创建 termios 结构体来保存串口配置
struct termios tty;
memset(&tty, 0, sizeof(tty));
if (tcgetattr(serial_port, &tty) != 0) {
printf("Error %i from tcgetattr: %s\n", errno, strerror(errno));
return 1;
}
// 设置波特率
cfsetispeed(&tty, B9600);
cfsetospeed(&tty, B9600);
// 设置数据位数
tty.c_cflag &= ~CSIZE; // 清除当前的设置
tty.c_cflag |= CS8; // 8位数据长度
// 设置奇偶校验位(可选)
tty.c_cflag &= ~PARENB;
// 设置停止位(1位停止位)
tty.c_cflag &= ~CSTOPB;
// 设置为非规范模式
tty.c_lflag &= ~ICANON;
tty.c_lflag &= ~ECHO; // 关闭回显
tty.c_lflag &= ~ECHOE; // 关闭回显擦除
tty.c_lflag &= ~ECHONL; // 关闭换行回显
// 设置读取等待时间和最小接收字符
tty.c_cc[VTIME] = 1; // 读取超时设置为10*0.1秒
tty.c_cc[VMIN] = 0;
// 保存串口设置
if (tcsetattr(serial_port, TCSANOW, &tty) != 0) {
printf("Error %i from tcsetattr: %s\n", errno, strerror(errno));
return 1;
}
// 写入串口数据
char write_buf[] = "Hello, serial port!";
write(serial_port, write_buf, sizeof(write_buf));
// 读取串口数据
char read_buf[256];
memset(&read_buf, '\0', sizeof(read_buf));
int num_bytes = read(serial_port, &read_buf, sizeof(read_buf));
if (num_bytes < 0) {
printf("Error reading: %s\n", strerror(errno));
return 1;
}
printf("Read %i bytes. Received message: %s\n", num_bytes, read_buf);
// 关闭串口
close(serial_port);
return 0;
}
```
阅读全文
相关推荐
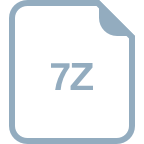
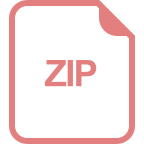
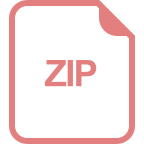
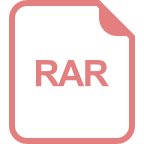
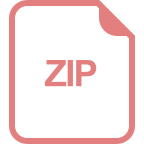
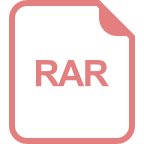
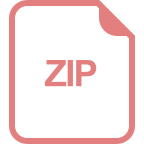
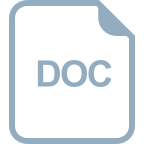
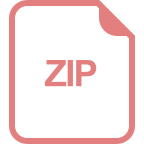
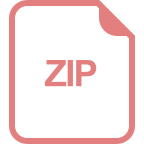
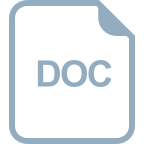
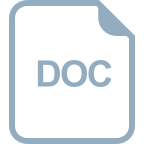
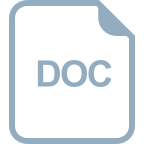
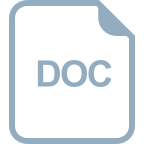

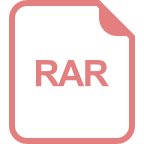