编写具有以下要求的完整程序: 1. 使用 5x5 bool二维数组来表示需要遍历的图形的连通性。 2. 使用深度优先算法遍历图形。 3. 使用广度优先算法遍历图形。 4、根据图的连通性合理选择遍历的起始位置。
时间: 2024-02-11 11:07:29 浏览: 77
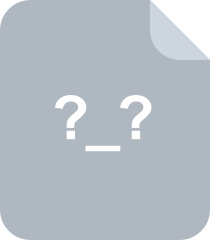
图的遍历算法:广度优先、深度优先

好的,下面是一个满足你需求的完整程序。该程序使用5x5 bool二维数组来表示需要遍历的图形的连通性,使用深度优先算法和广度优先算法遍历图形,并根据图的连通性合理选择遍历的起始位置。
```python
# 定义5x5 bool二维数组表示图形的连通性
graph = [
[False, True, False, True, False],
[True, False, True, False, True],
[False, True, False, True, False],
[True, False, True, False, True],
[False, True, False, True, False]
]
# 深度优先遍历算法
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start)
for i in range(len(graph)):
if graph[start][i] and i not in visited:
dfs(graph, i, visited)
# 广度优先遍历算法
def bfs(graph, start):
visited, queue = set(), [start]
visited.add(start)
while queue:
vertex = queue.pop(0)
print(vertex)
for i in range(len(graph)):
if graph[vertex][i] and i not in visited:
visited.add(i)
queue.append(i)
# 根据图的连通性合理选择遍历的起始位置
def choose_start(graph):
for i in range(len(graph)):
if True in graph[i]:
return i
return 0
# 主程序
if __name__ == '__main__':
start = choose_start(graph)
print("深度优先遍历结果:")
dfs(graph, start)
print("广度优先遍历结果:")
bfs(graph, start)
```
该程序定义了深度优先遍历算法和广度优先遍历算法,并使用5x5 bool二维数组表示需要遍历的图形的连通性。程序还根据图的连通性合理选择遍历的起始位置。
在主程序中,首先通过 choose_start 函数选择遍历的起始位置。然后,使用深度优先遍历算法和广度优先遍历算法遍历图形,并输出遍历结果。
阅读全文
相关推荐
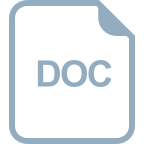





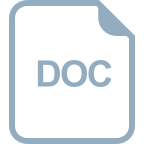

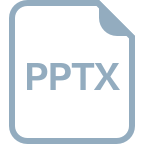
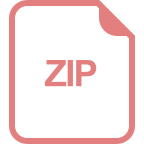
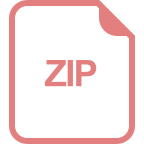
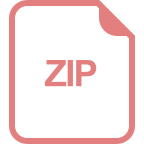
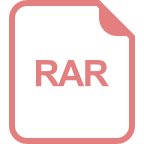
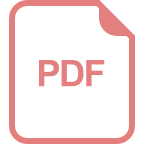


