请实现c加加的无锁队列的代码
时间: 2024-05-05 22:19:09 浏览: 117
好的,以下是一个简单的无锁队列的C++代码实现:
```c++
#include <atomic>
template<typename T>
class LockFreeQueue {
public:
LockFreeQueue() : head_(new Node), tail_(head_.load()) {}
~LockFreeQueue() {
while(head_ != nullptr) {
Node* temp = head_;
head_ = head_->next;
delete temp;
}
}
void push(const T& value) {
Node* node = new Node;
node->data = value;
Node* curr_tail = tail_.load();
curr_tail->next = node;
// atomic compare-and-swap operation for tail pointer
tail_.compare_exchange_strong(curr_tail, node);
}
bool pop(T& value) {
Node* curr_head = head_.load();
Node* curr_tail = tail_.load();
Node* curr_next = curr_head->next;
if (curr_head == curr_tail) {
return false;
}
// atomic compare-and-swap operation for head pointer
if (head_.compare_exchange_strong(curr_head, curr_next)) {
value = curr_next->data;
delete curr_head;
return true;
} else {
return false;
}
}
private:
struct Node {
T data;
Node* next{nullptr};
};
std::atomic<Node*> head_;
std::atomic<Node*> tail_;
};
```
这是一个简单的无锁队列的实现,使用了C++11的原子操作对head和tail指针进行操作,实现了线程安全的队列操作。在push操作中,我们创建一个新的节点,并通过原子操作将新的节点连接到当前的tail节点之后,而在pop操作中,我们通过原子操作将head指针后移一位,获得值后将该节点删除。由于这个实现是无锁的,因此可以在多个线程中安全地操作队列。
阅读全文
相关推荐
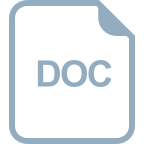
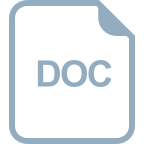
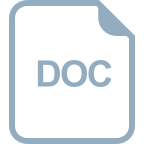















