使用JAVA程序编写代码,使用递归的思想编写函数factorial(int n) 计算 n的阶乘,并在main函数中调用factorial(int n) 输出5的阶乘
时间: 2024-09-19 11:10:53 浏览: 42
在Java中,你可以使用递归的方式来编写计算阶乘的函数。下面是一个简单的示例:
```java
public class FactorialExample {
public static long factorial(int n) {
// 基线条件:当n等于1时,返回1
if (n == 1) {
return 1;
}
// 递归条件:n的阶乘等于n乘以(n-1)的阶乘
else {
return n * factorial(n - 1);
}
}
public static void main(String[] args) {
// 调用factorial函数计算5的阶乘并打印结果
int n = 5;
long result = factorial(n);
System.out.println("The factorial of " + n + " is: " + result);
}
}
```
当你运行这个`main`函数时,它会输出`The factorial of 5 is: 120`,这是5的阶乘。
相关问题
用JAVA使用递归的思想编写函数 factorial(int n) 计算n的阶乘,并在main函数中调用 factorial(int n) 输出5的阶乘
在Java中,递归是一种通过函数自身调用自身来解决问题的技术。要编写计算阶乘的递归函数`factorial(int n)`,你可以按照下面的方式来完成:
```java
public class Main {
public static void main(String[] args) {
int number = 5;
int result = factorial(number);
System.out.println("The factorial of " + number + " is: " + result);
}
// 递归函数,用于计算阶乘
public static int factorial(int n) {
if (n == 0 || n == 1) { // 阶乘的基本情况,0和1的阶乘都是1
return 1;
} else {
return n * factorial(n - 1); // 递归调用,n! = n * (n-1)!
}
}
}
```
在这个例子中,我们首先检查基本情况,当n等于0或1时返回1(因为0和1的阶乘都是1)。如果n不是0或1,我们就递归地调用`factorial(n - 1)`并将结果乘以n,直到达到基本情况为止。
编写递归函数int factorial(int n)计算n(0<=n<=10)的阶乘,在该函数中用return语句返回n的阶乘的值。 注意: (1)不要修改函数名、函数返回类型、参数个数、参数名和参数类型。 (2)不能使用for、while循环语句和goto语句。
下面是一个使用递归函数计算整数 n 阶乘的示例代码:
```c++
#include <iostream>
using namespace std;
int factorial(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
int main() {
int n;
cin >> n;
cout << factorial(n) << endl;
return 0;
}
```
这段代码中,`factorial(int n)` 函数是一个递归函数,用于计算整数 n 的阶乘。当 n 等于 0 时,函数返回 1;否则,函数返回 n 与 `factorial(n - 1)` 的乘积。在主函数中,从键盘输入 n 的值,然后调用 `factorial(n)` 函数计算 n 的阶乘,并将结果输出到屏幕上。
这道题目的要求是不使用循环语句和 goto 语句,因此使用递归函数计算阶乘是一个不错的选择。递归函数的本质是一种自我调用的函数,通过不断调用自身来实现计算。在这个例子中,当 n 等于 0 时,递归函数会停止调用自身,返回 1;否则,递归函数会继续调用自身,直到 n 等于 0 为止。
阅读全文
相关推荐
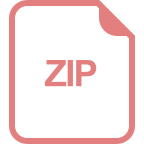
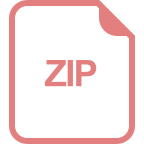
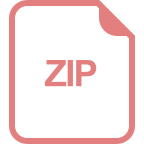













