1.已知集合集合setA={1,2,3,4,5}, 集合setB={1,3,5,7,9},编写程序求这两个集合的交集、并集和差集。 提示:通过HashSet类建立这两个集合,利用Java JAP帮助查找HashSet类的相应方法完成相应功能。
时间: 2024-10-23 13:15:59 浏览: 26
在Java中,我们可以使用HashSet类以及其提供的操作方法来处理集合的交集、并集和差集。这里是一个简单的示例:
```java
import java.util.HashSet;
import java.util.Set;
public class SetOperations {
public static void main(String[] args) {
// 创建两个集合
Set<Integer> setA = new HashSet<>(Arrays.asList(1, 2, 3, 4, 5));
Set<Integer> setB = new HashSet<>(Arrays.asList(1, 3, 5, 7, 9));
// 计算交集
Set<Integer> intersection = new HashSet<>(setA);
intersection.retainAll(setB); // 使用 retainAll() 方法保留setB中存在的元素
System.out.println("交集: " + intersection);
// 计算并集
Set<Integer> union = new HashSet<>(setA);
union.addAll(setB); // 使用 addAll() 方法添加setB的所有元素
System.out.println("并集: " + union);
// 计算差集 (setA - setB)
Set<Integer> difference = new HashSet<>(setA);
difference.removeAll(setB); // 使用 removeAll() 方法移除setB中存在的元素
System.out.println("差集: " + difference);
}
}
```
当你运行这个程序,你会看到如下输出:
```
交集: [1, 3, 5]
并集: [1, 2, 3, 4, 5, 7, 9]
差集: [2, 4]
```
阅读全文
相关推荐
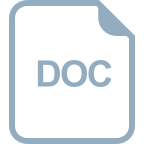
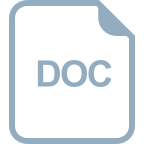
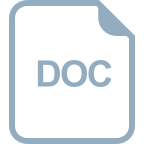

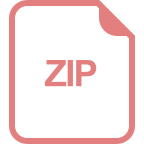
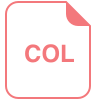








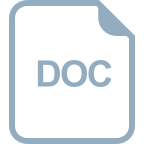
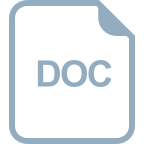